Convert object array to hash map using lodash
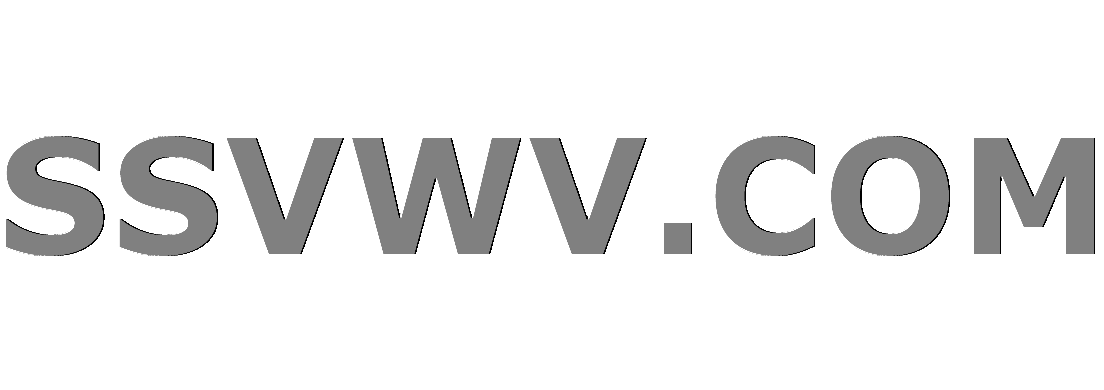
Multi tool use
The use case is to convert an array of objects into a hash map where one property is the key and the other property is the value. Common case of using this is converting a "link" object in a hypermedia response into a hash map of links.
jsfiddle
function toHashMap(data, name, value) {
return _.zipObject(_.pluck(data, name),
_.pluck(data, value));
}
function toMap(data, name, value) {
return _.reduce(data, function(acc, item) {
acc[item[name]] = item[value];
return acc;
}, {});
}
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
console.log(toHashMap(data, 'rel', 'href'));
console.log(toMap(data, 'rel', 'href'));
toHashMap
appears much more readable, but less efficient. toMap
seems to have the best efficiency at the expense of readability.
Is there a more elegant solution - i.e. both readable and efficient? I suspect that Lodash might have a function to do this already, but I haven't found anything like this after looking at the API documentation.
javascript beginner lodash.js
add a comment |
The use case is to convert an array of objects into a hash map where one property is the key and the other property is the value. Common case of using this is converting a "link" object in a hypermedia response into a hash map of links.
jsfiddle
function toHashMap(data, name, value) {
return _.zipObject(_.pluck(data, name),
_.pluck(data, value));
}
function toMap(data, name, value) {
return _.reduce(data, function(acc, item) {
acc[item[name]] = item[value];
return acc;
}, {});
}
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
console.log(toHashMap(data, 'rel', 'href'));
console.log(toMap(data, 'rel', 'href'));
toHashMap
appears much more readable, but less efficient. toMap
seems to have the best efficiency at the expense of readability.
Is there a more elegant solution - i.e. both readable and efficient? I suspect that Lodash might have a function to do this already, but I haven't found anything like this after looking at the API documentation.
javascript beginner lodash.js
I've compiled the most common ways to turn an object into an array in this lodash feature request (which currently needs upvotes!)
– BlueRaja - Danny Pflughoeft
Nov 17 '16 at 23:10
add a comment |
The use case is to convert an array of objects into a hash map where one property is the key and the other property is the value. Common case of using this is converting a "link" object in a hypermedia response into a hash map of links.
jsfiddle
function toHashMap(data, name, value) {
return _.zipObject(_.pluck(data, name),
_.pluck(data, value));
}
function toMap(data, name, value) {
return _.reduce(data, function(acc, item) {
acc[item[name]] = item[value];
return acc;
}, {});
}
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
console.log(toHashMap(data, 'rel', 'href'));
console.log(toMap(data, 'rel', 'href'));
toHashMap
appears much more readable, but less efficient. toMap
seems to have the best efficiency at the expense of readability.
Is there a more elegant solution - i.e. both readable and efficient? I suspect that Lodash might have a function to do this already, but I haven't found anything like this after looking at the API documentation.
javascript beginner lodash.js
The use case is to convert an array of objects into a hash map where one property is the key and the other property is the value. Common case of using this is converting a "link" object in a hypermedia response into a hash map of links.
jsfiddle
function toHashMap(data, name, value) {
return _.zipObject(_.pluck(data, name),
_.pluck(data, value));
}
function toMap(data, name, value) {
return _.reduce(data, function(acc, item) {
acc[item[name]] = item[value];
return acc;
}, {});
}
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
console.log(toHashMap(data, 'rel', 'href'));
console.log(toMap(data, 'rel', 'href'));
toHashMap
appears much more readable, but less efficient. toMap
seems to have the best efficiency at the expense of readability.
Is there a more elegant solution - i.e. both readable and efficient? I suspect that Lodash might have a function to do this already, but I haven't found anything like this after looking at the API documentation.
javascript beginner lodash.js
javascript beginner lodash.js
edited Mar 24 '16 at 11:00


200_success
129k15152414
129k15152414
asked Jul 21 '14 at 20:01
PetePete
1,29921019
1,29921019
I've compiled the most common ways to turn an object into an array in this lodash feature request (which currently needs upvotes!)
– BlueRaja - Danny Pflughoeft
Nov 17 '16 at 23:10
add a comment |
I've compiled the most common ways to turn an object into an array in this lodash feature request (which currently needs upvotes!)
– BlueRaja - Danny Pflughoeft
Nov 17 '16 at 23:10
I've compiled the most common ways to turn an object into an array in this lodash feature request (which currently needs upvotes!)
– BlueRaja - Danny Pflughoeft
Nov 17 '16 at 23:10
I've compiled the most common ways to turn an object into an array in this lodash feature request (which currently needs upvotes!)
– BlueRaja - Danny Pflughoeft
Nov 17 '16 at 23:10
add a comment |
7 Answers
7
active
oldest
votes
I think you are looking for _.keyBy
(or _.indexBy
in older versions)
_.keyBy(data, 'rel');
3
I don't understand why is this the accepted answer since it doesn't give the same result as the exposed functions
– Pau Fracés
Feb 17 '15 at 15:53
1
@PauFracés See below for revised solution.
– Pete
Mar 13 '15 at 21:14
4
Note: Now known as_.keyBy
(lodash.com/docs#keyBy).
– jtheletter
Feb 18 '16 at 2:07
add a comment |
Revised Solution
As per Pau Fracés comment above, here is the complete solution. The solution given by John Anderson would index all objects by the key. However, this would not create a key-value pair map.
To complete the solution of generating a full hash map, the values must be mapped to the key. Using the transform function, the values can be extracted from the objects and mapped back to the key or in this case rel
.
Pseudo Code
- Index all objects by the chosen key.
- Map all values to the key.
Code
Below is the complete code with logging enabled. For a non-logging version, remove all lines with the tap
function.
var data = [{ rel: 'link1', href: 'url1' },
{ rel: 'link2', href: 'url2' },
{ rel: 'link3', href: 'url3' },
{ rel: 'link4', href: 'url4' }];
function log(value) {
document.getElementById("output").innerHTML += JSON.stringify(value, null, 2) + "n"
}
var hashmap = _.chain(data)
.keyBy('rel')
.tap(log) // Line used just for logging
.mapValues('href')
.tap(log)
.value();
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.6.1/lodash.min.js"></script>
<pre id="output"></pre>
1
Nice solution! Personally I would go formapValues
instead oftransform
. Withtransform
you're updating state outside the closure (it is a side-effecting function), whereas withmapValues
you are not.
– Martijn
Jul 7 '15 at 9:14
1
@Martijn Oh great suggestion! Makes for a much cleaner solution IMHO. I've updated the solution with your suggestion. Thanks!
– Pete
Jul 10 '15 at 20:21
1
@Pete Awesome! Just realized that lodash v4 renamedindexBy
tokeyBy
– Pau Fracés
Feb 11 '16 at 20:22
Updated to usekeyBy
– Pete
Mar 8 '16 at 13:18
1
with lodash-fp this can also be done as a one-linerflow(fpKeyBy('rel'), fpMapValues('href'))(data)
(fp-prefixed functions are fp-lodash versions)
– Spain Train
May 7 '16 at 0:05
add a comment |
In ES7 it's now as simple as:
data.reduce((acc, { rel, href }) => ({ ...acc, [rel]: href }), {});
(without even using Lodash).
would be great if it worked. but it doesn't seem to work! (node 5.0.0)
– Rene Wooller
Sep 8 '16 at 0:55
This is ES7, not ES2015
– Rene Wooller
Sep 8 '16 at 1:01
@ReneWooller Right, sorry. Hard to keep track of what's what when using Babel :)
– hon2a
Sep 8 '16 at 9:15
That works, and it's nice to not have to have to have the lodash dependency, but damn if the lodash way isn't a million times more readable.
– Thor84no
Jan 29 '18 at 10:31
@Thor84no Full Lodash solution is e.g._.mapValues(_.keyBy(data, 'rel'), 'href')
(the accepted answer doesn't produce what the OP asked for). Readability depends on your familiarity with Lodash though.
– hon2a
Jan 29 '18 at 13:56
|
show 2 more comments
A simpler way would be to use "reduce".
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
var hashmap = _.reduce(data, function(hash, value) {
var key = value['rel'];
hash[key] = value['href'];
return hash;
}, {});
JSFiddle: https://jsfiddle.net/6txzzxq2/
With reduce you can iterate over the source array with an "accumulator" (in this case, a new object). Above we key the hash by the rel attribute with href as the value.
You could also use.transform
to reduce the amount of code... but that's a matter of personal preference.
– Pete
Mar 14 '16 at 15:22
As stated by Martijn in a previous comment, both.reduce
and.transform
update state outside the closure whereasmapValues
does not. So keep this in mind when using.reduce
.
– Pete
Mar 16 '16 at 21:37
@Pete Updating outside state is not an inherent property of.reduce
, but rather of the reducer itself. You can just as well use.reduce
without side-effects (see my short answer).
– hon2a
Mar 24 '16 at 10:42
@hon2a True... I one thing that bugs me about this particularreduce
solution, though, is that 'rel' and 'href' are hardcoded into the callback. It makes it less reusable. I tried to avoid that in the original solution. The maintenance cost of a one-liner to a five-liner is much more appealing to me. As for readability,reduce
is less fluent comparedkeyBy
andmapValues
to a non-programmer. Granted, all this is at the cost of performance and so it's not the end all of solutions. Thanks for updating to ES2015.
– Pete
Mar 24 '16 at 13:40
@Pete I'd argue that a simple use ofreduce
is actually better readable thankeyBy
+mapValues(propNameShortcut)
, asreduce
is a native (thus ubiquitous) method that doesn't require you to know the details of a specific (Lodash) API.
– hon2a
Apr 11 '16 at 10:39
|
show 2 more comments
Since lodash 4, you can use _.fromPairs:
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
var hashmap = _.fromPairs(data.map(function(item) {
return [item.rel, item.href];
}));
1
Is there a way to parameterize 'rel' and 'href' outside of themap
method to make the solution more generic?
– Pete
May 25 '16 at 14:21
1
function makePair(keyProperty, valueProperty) { return function(item) { return [item[keyProperty], item[valueProperty]]; }; } var hashmap = _.fromPairs(data.map(makePair('rel', 'href')));
– Ricardo Stuven
Aug 28 '17 at 13:20
add a comment |
With Lodash 4:
Using keyBy
and mapValues
_.mapValues(_.keyBy(data, 'rel'), v => v.href);
Or:
_.chain(data).keyBy('rel').mapValues(v => v.href).value();
add a comment |
I see a lot of good answers already. I would just like to add one more answer to the list using Ramda's indexBy.
const data = [{
id: 1,
foo: 'bar'
},
{
id: 2,
baz: 'lorem'
}
];
const dataById = R.indexBy(R.prop('id'), data);
console.log(dataById)
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.25.0/ramda.min.js"></script>
I hope it helps someone else :)
This isn't really a review of the code provided, is it?
– Mast
Jan 15 '18 at 20:20
Sorry misunderstood the use of this website. More familiar with StackOverflow :)
– Abhishek Ghosh
Jan 16 '18 at 0:33
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f57614%2fconvert-object-array-to-hash-map-using-lodash%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
7 Answers
7
active
oldest
votes
7 Answers
7
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think you are looking for _.keyBy
(or _.indexBy
in older versions)
_.keyBy(data, 'rel');
3
I don't understand why is this the accepted answer since it doesn't give the same result as the exposed functions
– Pau Fracés
Feb 17 '15 at 15:53
1
@PauFracés See below for revised solution.
– Pete
Mar 13 '15 at 21:14
4
Note: Now known as_.keyBy
(lodash.com/docs#keyBy).
– jtheletter
Feb 18 '16 at 2:07
add a comment |
I think you are looking for _.keyBy
(or _.indexBy
in older versions)
_.keyBy(data, 'rel');
3
I don't understand why is this the accepted answer since it doesn't give the same result as the exposed functions
– Pau Fracés
Feb 17 '15 at 15:53
1
@PauFracés See below for revised solution.
– Pete
Mar 13 '15 at 21:14
4
Note: Now known as_.keyBy
(lodash.com/docs#keyBy).
– jtheletter
Feb 18 '16 at 2:07
add a comment |
I think you are looking for _.keyBy
(or _.indexBy
in older versions)
_.keyBy(data, 'rel');
I think you are looking for _.keyBy
(or _.indexBy
in older versions)
_.keyBy(data, 'rel');
edited Jun 8 '16 at 3:34
leedm777
1033
1033
answered Jul 21 '14 at 20:14
Jon AndersonJon Anderson
1,00972
1,00972
3
I don't understand why is this the accepted answer since it doesn't give the same result as the exposed functions
– Pau Fracés
Feb 17 '15 at 15:53
1
@PauFracés See below for revised solution.
– Pete
Mar 13 '15 at 21:14
4
Note: Now known as_.keyBy
(lodash.com/docs#keyBy).
– jtheletter
Feb 18 '16 at 2:07
add a comment |
3
I don't understand why is this the accepted answer since it doesn't give the same result as the exposed functions
– Pau Fracés
Feb 17 '15 at 15:53
1
@PauFracés See below for revised solution.
– Pete
Mar 13 '15 at 21:14
4
Note: Now known as_.keyBy
(lodash.com/docs#keyBy).
– jtheletter
Feb 18 '16 at 2:07
3
3
I don't understand why is this the accepted answer since it doesn't give the same result as the exposed functions
– Pau Fracés
Feb 17 '15 at 15:53
I don't understand why is this the accepted answer since it doesn't give the same result as the exposed functions
– Pau Fracés
Feb 17 '15 at 15:53
1
1
@PauFracés See below for revised solution.
– Pete
Mar 13 '15 at 21:14
@PauFracés See below for revised solution.
– Pete
Mar 13 '15 at 21:14
4
4
Note: Now known as
_.keyBy
(lodash.com/docs#keyBy).– jtheletter
Feb 18 '16 at 2:07
Note: Now known as
_.keyBy
(lodash.com/docs#keyBy).– jtheletter
Feb 18 '16 at 2:07
add a comment |
Revised Solution
As per Pau Fracés comment above, here is the complete solution. The solution given by John Anderson would index all objects by the key. However, this would not create a key-value pair map.
To complete the solution of generating a full hash map, the values must be mapped to the key. Using the transform function, the values can be extracted from the objects and mapped back to the key or in this case rel
.
Pseudo Code
- Index all objects by the chosen key.
- Map all values to the key.
Code
Below is the complete code with logging enabled. For a non-logging version, remove all lines with the tap
function.
var data = [{ rel: 'link1', href: 'url1' },
{ rel: 'link2', href: 'url2' },
{ rel: 'link3', href: 'url3' },
{ rel: 'link4', href: 'url4' }];
function log(value) {
document.getElementById("output").innerHTML += JSON.stringify(value, null, 2) + "n"
}
var hashmap = _.chain(data)
.keyBy('rel')
.tap(log) // Line used just for logging
.mapValues('href')
.tap(log)
.value();
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.6.1/lodash.min.js"></script>
<pre id="output"></pre>
1
Nice solution! Personally I would go formapValues
instead oftransform
. Withtransform
you're updating state outside the closure (it is a side-effecting function), whereas withmapValues
you are not.
– Martijn
Jul 7 '15 at 9:14
1
@Martijn Oh great suggestion! Makes for a much cleaner solution IMHO. I've updated the solution with your suggestion. Thanks!
– Pete
Jul 10 '15 at 20:21
1
@Pete Awesome! Just realized that lodash v4 renamedindexBy
tokeyBy
– Pau Fracés
Feb 11 '16 at 20:22
Updated to usekeyBy
– Pete
Mar 8 '16 at 13:18
1
with lodash-fp this can also be done as a one-linerflow(fpKeyBy('rel'), fpMapValues('href'))(data)
(fp-prefixed functions are fp-lodash versions)
– Spain Train
May 7 '16 at 0:05
add a comment |
Revised Solution
As per Pau Fracés comment above, here is the complete solution. The solution given by John Anderson would index all objects by the key. However, this would not create a key-value pair map.
To complete the solution of generating a full hash map, the values must be mapped to the key. Using the transform function, the values can be extracted from the objects and mapped back to the key or in this case rel
.
Pseudo Code
- Index all objects by the chosen key.
- Map all values to the key.
Code
Below is the complete code with logging enabled. For a non-logging version, remove all lines with the tap
function.
var data = [{ rel: 'link1', href: 'url1' },
{ rel: 'link2', href: 'url2' },
{ rel: 'link3', href: 'url3' },
{ rel: 'link4', href: 'url4' }];
function log(value) {
document.getElementById("output").innerHTML += JSON.stringify(value, null, 2) + "n"
}
var hashmap = _.chain(data)
.keyBy('rel')
.tap(log) // Line used just for logging
.mapValues('href')
.tap(log)
.value();
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.6.1/lodash.min.js"></script>
<pre id="output"></pre>
1
Nice solution! Personally I would go formapValues
instead oftransform
. Withtransform
you're updating state outside the closure (it is a side-effecting function), whereas withmapValues
you are not.
– Martijn
Jul 7 '15 at 9:14
1
@Martijn Oh great suggestion! Makes for a much cleaner solution IMHO. I've updated the solution with your suggestion. Thanks!
– Pete
Jul 10 '15 at 20:21
1
@Pete Awesome! Just realized that lodash v4 renamedindexBy
tokeyBy
– Pau Fracés
Feb 11 '16 at 20:22
Updated to usekeyBy
– Pete
Mar 8 '16 at 13:18
1
with lodash-fp this can also be done as a one-linerflow(fpKeyBy('rel'), fpMapValues('href'))(data)
(fp-prefixed functions are fp-lodash versions)
– Spain Train
May 7 '16 at 0:05
add a comment |
Revised Solution
As per Pau Fracés comment above, here is the complete solution. The solution given by John Anderson would index all objects by the key. However, this would not create a key-value pair map.
To complete the solution of generating a full hash map, the values must be mapped to the key. Using the transform function, the values can be extracted from the objects and mapped back to the key or in this case rel
.
Pseudo Code
- Index all objects by the chosen key.
- Map all values to the key.
Code
Below is the complete code with logging enabled. For a non-logging version, remove all lines with the tap
function.
var data = [{ rel: 'link1', href: 'url1' },
{ rel: 'link2', href: 'url2' },
{ rel: 'link3', href: 'url3' },
{ rel: 'link4', href: 'url4' }];
function log(value) {
document.getElementById("output").innerHTML += JSON.stringify(value, null, 2) + "n"
}
var hashmap = _.chain(data)
.keyBy('rel')
.tap(log) // Line used just for logging
.mapValues('href')
.tap(log)
.value();
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.6.1/lodash.min.js"></script>
<pre id="output"></pre>
Revised Solution
As per Pau Fracés comment above, here is the complete solution. The solution given by John Anderson would index all objects by the key. However, this would not create a key-value pair map.
To complete the solution of generating a full hash map, the values must be mapped to the key. Using the transform function, the values can be extracted from the objects and mapped back to the key or in this case rel
.
Pseudo Code
- Index all objects by the chosen key.
- Map all values to the key.
Code
Below is the complete code with logging enabled. For a non-logging version, remove all lines with the tap
function.
var data = [{ rel: 'link1', href: 'url1' },
{ rel: 'link2', href: 'url2' },
{ rel: 'link3', href: 'url3' },
{ rel: 'link4', href: 'url4' }];
function log(value) {
document.getElementById("output").innerHTML += JSON.stringify(value, null, 2) + "n"
}
var hashmap = _.chain(data)
.keyBy('rel')
.tap(log) // Line used just for logging
.mapValues('href')
.tap(log)
.value();
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.6.1/lodash.min.js"></script>
<pre id="output"></pre>
var data = [{ rel: 'link1', href: 'url1' },
{ rel: 'link2', href: 'url2' },
{ rel: 'link3', href: 'url3' },
{ rel: 'link4', href: 'url4' }];
function log(value) {
document.getElementById("output").innerHTML += JSON.stringify(value, null, 2) + "n"
}
var hashmap = _.chain(data)
.keyBy('rel')
.tap(log) // Line used just for logging
.mapValues('href')
.tap(log)
.value();
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.6.1/lodash.min.js"></script>
<pre id="output"></pre>
var data = [{ rel: 'link1', href: 'url1' },
{ rel: 'link2', href: 'url2' },
{ rel: 'link3', href: 'url3' },
{ rel: 'link4', href: 'url4' }];
function log(value) {
document.getElementById("output").innerHTML += JSON.stringify(value, null, 2) + "n"
}
var hashmap = _.chain(data)
.keyBy('rel')
.tap(log) // Line used just for logging
.mapValues('href')
.tap(log)
.value();
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.6.1/lodash.min.js"></script>
<pre id="output"></pre>
edited Mar 8 '16 at 15:40
answered Mar 13 '15 at 18:13
PetePete
1,29921019
1,29921019
1
Nice solution! Personally I would go formapValues
instead oftransform
. Withtransform
you're updating state outside the closure (it is a side-effecting function), whereas withmapValues
you are not.
– Martijn
Jul 7 '15 at 9:14
1
@Martijn Oh great suggestion! Makes for a much cleaner solution IMHO. I've updated the solution with your suggestion. Thanks!
– Pete
Jul 10 '15 at 20:21
1
@Pete Awesome! Just realized that lodash v4 renamedindexBy
tokeyBy
– Pau Fracés
Feb 11 '16 at 20:22
Updated to usekeyBy
– Pete
Mar 8 '16 at 13:18
1
with lodash-fp this can also be done as a one-linerflow(fpKeyBy('rel'), fpMapValues('href'))(data)
(fp-prefixed functions are fp-lodash versions)
– Spain Train
May 7 '16 at 0:05
add a comment |
1
Nice solution! Personally I would go formapValues
instead oftransform
. Withtransform
you're updating state outside the closure (it is a side-effecting function), whereas withmapValues
you are not.
– Martijn
Jul 7 '15 at 9:14
1
@Martijn Oh great suggestion! Makes for a much cleaner solution IMHO. I've updated the solution with your suggestion. Thanks!
– Pete
Jul 10 '15 at 20:21
1
@Pete Awesome! Just realized that lodash v4 renamedindexBy
tokeyBy
– Pau Fracés
Feb 11 '16 at 20:22
Updated to usekeyBy
– Pete
Mar 8 '16 at 13:18
1
with lodash-fp this can also be done as a one-linerflow(fpKeyBy('rel'), fpMapValues('href'))(data)
(fp-prefixed functions are fp-lodash versions)
– Spain Train
May 7 '16 at 0:05
1
1
Nice solution! Personally I would go for
mapValues
instead of transform
. With transform
you're updating state outside the closure (it is a side-effecting function), whereas with mapValues
you are not.– Martijn
Jul 7 '15 at 9:14
Nice solution! Personally I would go for
mapValues
instead of transform
. With transform
you're updating state outside the closure (it is a side-effecting function), whereas with mapValues
you are not.– Martijn
Jul 7 '15 at 9:14
1
1
@Martijn Oh great suggestion! Makes for a much cleaner solution IMHO. I've updated the solution with your suggestion. Thanks!
– Pete
Jul 10 '15 at 20:21
@Martijn Oh great suggestion! Makes for a much cleaner solution IMHO. I've updated the solution with your suggestion. Thanks!
– Pete
Jul 10 '15 at 20:21
1
1
@Pete Awesome! Just realized that lodash v4 renamed
indexBy
to keyBy
– Pau Fracés
Feb 11 '16 at 20:22
@Pete Awesome! Just realized that lodash v4 renamed
indexBy
to keyBy
– Pau Fracés
Feb 11 '16 at 20:22
Updated to use
keyBy
– Pete
Mar 8 '16 at 13:18
Updated to use
keyBy
– Pete
Mar 8 '16 at 13:18
1
1
with lodash-fp this can also be done as a one-liner
flow(fpKeyBy('rel'), fpMapValues('href'))(data)
(fp-prefixed functions are fp-lodash versions)– Spain Train
May 7 '16 at 0:05
with lodash-fp this can also be done as a one-liner
flow(fpKeyBy('rel'), fpMapValues('href'))(data)
(fp-prefixed functions are fp-lodash versions)– Spain Train
May 7 '16 at 0:05
add a comment |
In ES7 it's now as simple as:
data.reduce((acc, { rel, href }) => ({ ...acc, [rel]: href }), {});
(without even using Lodash).
would be great if it worked. but it doesn't seem to work! (node 5.0.0)
– Rene Wooller
Sep 8 '16 at 0:55
This is ES7, not ES2015
– Rene Wooller
Sep 8 '16 at 1:01
@ReneWooller Right, sorry. Hard to keep track of what's what when using Babel :)
– hon2a
Sep 8 '16 at 9:15
That works, and it's nice to not have to have to have the lodash dependency, but damn if the lodash way isn't a million times more readable.
– Thor84no
Jan 29 '18 at 10:31
@Thor84no Full Lodash solution is e.g._.mapValues(_.keyBy(data, 'rel'), 'href')
(the accepted answer doesn't produce what the OP asked for). Readability depends on your familiarity with Lodash though.
– hon2a
Jan 29 '18 at 13:56
|
show 2 more comments
In ES7 it's now as simple as:
data.reduce((acc, { rel, href }) => ({ ...acc, [rel]: href }), {});
(without even using Lodash).
would be great if it worked. but it doesn't seem to work! (node 5.0.0)
– Rene Wooller
Sep 8 '16 at 0:55
This is ES7, not ES2015
– Rene Wooller
Sep 8 '16 at 1:01
@ReneWooller Right, sorry. Hard to keep track of what's what when using Babel :)
– hon2a
Sep 8 '16 at 9:15
That works, and it's nice to not have to have to have the lodash dependency, but damn if the lodash way isn't a million times more readable.
– Thor84no
Jan 29 '18 at 10:31
@Thor84no Full Lodash solution is e.g._.mapValues(_.keyBy(data, 'rel'), 'href')
(the accepted answer doesn't produce what the OP asked for). Readability depends on your familiarity with Lodash though.
– hon2a
Jan 29 '18 at 13:56
|
show 2 more comments
In ES7 it's now as simple as:
data.reduce((acc, { rel, href }) => ({ ...acc, [rel]: href }), {});
(without even using Lodash).
In ES7 it's now as simple as:
data.reduce((acc, { rel, href }) => ({ ...acc, [rel]: href }), {});
(without even using Lodash).
edited 17 hours ago
answered Mar 24 '16 at 10:38
hon2ahon2a
28137
28137
would be great if it worked. but it doesn't seem to work! (node 5.0.0)
– Rene Wooller
Sep 8 '16 at 0:55
This is ES7, not ES2015
– Rene Wooller
Sep 8 '16 at 1:01
@ReneWooller Right, sorry. Hard to keep track of what's what when using Babel :)
– hon2a
Sep 8 '16 at 9:15
That works, and it's nice to not have to have to have the lodash dependency, but damn if the lodash way isn't a million times more readable.
– Thor84no
Jan 29 '18 at 10:31
@Thor84no Full Lodash solution is e.g._.mapValues(_.keyBy(data, 'rel'), 'href')
(the accepted answer doesn't produce what the OP asked for). Readability depends on your familiarity with Lodash though.
– hon2a
Jan 29 '18 at 13:56
|
show 2 more comments
would be great if it worked. but it doesn't seem to work! (node 5.0.0)
– Rene Wooller
Sep 8 '16 at 0:55
This is ES7, not ES2015
– Rene Wooller
Sep 8 '16 at 1:01
@ReneWooller Right, sorry. Hard to keep track of what's what when using Babel :)
– hon2a
Sep 8 '16 at 9:15
That works, and it's nice to not have to have to have the lodash dependency, but damn if the lodash way isn't a million times more readable.
– Thor84no
Jan 29 '18 at 10:31
@Thor84no Full Lodash solution is e.g._.mapValues(_.keyBy(data, 'rel'), 'href')
(the accepted answer doesn't produce what the OP asked for). Readability depends on your familiarity with Lodash though.
– hon2a
Jan 29 '18 at 13:56
would be great if it worked. but it doesn't seem to work! (node 5.0.0)
– Rene Wooller
Sep 8 '16 at 0:55
would be great if it worked. but it doesn't seem to work! (node 5.0.0)
– Rene Wooller
Sep 8 '16 at 0:55
This is ES7, not ES2015
– Rene Wooller
Sep 8 '16 at 1:01
This is ES7, not ES2015
– Rene Wooller
Sep 8 '16 at 1:01
@ReneWooller Right, sorry. Hard to keep track of what's what when using Babel :)
– hon2a
Sep 8 '16 at 9:15
@ReneWooller Right, sorry. Hard to keep track of what's what when using Babel :)
– hon2a
Sep 8 '16 at 9:15
That works, and it's nice to not have to have to have the lodash dependency, but damn if the lodash way isn't a million times more readable.
– Thor84no
Jan 29 '18 at 10:31
That works, and it's nice to not have to have to have the lodash dependency, but damn if the lodash way isn't a million times more readable.
– Thor84no
Jan 29 '18 at 10:31
@Thor84no Full Lodash solution is e.g.
_.mapValues(_.keyBy(data, 'rel'), 'href')
(the accepted answer doesn't produce what the OP asked for). Readability depends on your familiarity with Lodash though.– hon2a
Jan 29 '18 at 13:56
@Thor84no Full Lodash solution is e.g.
_.mapValues(_.keyBy(data, 'rel'), 'href')
(the accepted answer doesn't produce what the OP asked for). Readability depends on your familiarity with Lodash though.– hon2a
Jan 29 '18 at 13:56
|
show 2 more comments
A simpler way would be to use "reduce".
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
var hashmap = _.reduce(data, function(hash, value) {
var key = value['rel'];
hash[key] = value['href'];
return hash;
}, {});
JSFiddle: https://jsfiddle.net/6txzzxq2/
With reduce you can iterate over the source array with an "accumulator" (in this case, a new object). Above we key the hash by the rel attribute with href as the value.
You could also use.transform
to reduce the amount of code... but that's a matter of personal preference.
– Pete
Mar 14 '16 at 15:22
As stated by Martijn in a previous comment, both.reduce
and.transform
update state outside the closure whereasmapValues
does not. So keep this in mind when using.reduce
.
– Pete
Mar 16 '16 at 21:37
@Pete Updating outside state is not an inherent property of.reduce
, but rather of the reducer itself. You can just as well use.reduce
without side-effects (see my short answer).
– hon2a
Mar 24 '16 at 10:42
@hon2a True... I one thing that bugs me about this particularreduce
solution, though, is that 'rel' and 'href' are hardcoded into the callback. It makes it less reusable. I tried to avoid that in the original solution. The maintenance cost of a one-liner to a five-liner is much more appealing to me. As for readability,reduce
is less fluent comparedkeyBy
andmapValues
to a non-programmer. Granted, all this is at the cost of performance and so it's not the end all of solutions. Thanks for updating to ES2015.
– Pete
Mar 24 '16 at 13:40
@Pete I'd argue that a simple use ofreduce
is actually better readable thankeyBy
+mapValues(propNameShortcut)
, asreduce
is a native (thus ubiquitous) method that doesn't require you to know the details of a specific (Lodash) API.
– hon2a
Apr 11 '16 at 10:39
|
show 2 more comments
A simpler way would be to use "reduce".
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
var hashmap = _.reduce(data, function(hash, value) {
var key = value['rel'];
hash[key] = value['href'];
return hash;
}, {});
JSFiddle: https://jsfiddle.net/6txzzxq2/
With reduce you can iterate over the source array with an "accumulator" (in this case, a new object). Above we key the hash by the rel attribute with href as the value.
You could also use.transform
to reduce the amount of code... but that's a matter of personal preference.
– Pete
Mar 14 '16 at 15:22
As stated by Martijn in a previous comment, both.reduce
and.transform
update state outside the closure whereasmapValues
does not. So keep this in mind when using.reduce
.
– Pete
Mar 16 '16 at 21:37
@Pete Updating outside state is not an inherent property of.reduce
, but rather of the reducer itself. You can just as well use.reduce
without side-effects (see my short answer).
– hon2a
Mar 24 '16 at 10:42
@hon2a True... I one thing that bugs me about this particularreduce
solution, though, is that 'rel' and 'href' are hardcoded into the callback. It makes it less reusable. I tried to avoid that in the original solution. The maintenance cost of a one-liner to a five-liner is much more appealing to me. As for readability,reduce
is less fluent comparedkeyBy
andmapValues
to a non-programmer. Granted, all this is at the cost of performance and so it's not the end all of solutions. Thanks for updating to ES2015.
– Pete
Mar 24 '16 at 13:40
@Pete I'd argue that a simple use ofreduce
is actually better readable thankeyBy
+mapValues(propNameShortcut)
, asreduce
is a native (thus ubiquitous) method that doesn't require you to know the details of a specific (Lodash) API.
– hon2a
Apr 11 '16 at 10:39
|
show 2 more comments
A simpler way would be to use "reduce".
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
var hashmap = _.reduce(data, function(hash, value) {
var key = value['rel'];
hash[key] = value['href'];
return hash;
}, {});
JSFiddle: https://jsfiddle.net/6txzzxq2/
With reduce you can iterate over the source array with an "accumulator" (in this case, a new object). Above we key the hash by the rel attribute with href as the value.
A simpler way would be to use "reduce".
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
var hashmap = _.reduce(data, function(hash, value) {
var key = value['rel'];
hash[key] = value['href'];
return hash;
}, {});
JSFiddle: https://jsfiddle.net/6txzzxq2/
With reduce you can iterate over the source array with an "accumulator" (in this case, a new object). Above we key the hash by the rel attribute with href as the value.
answered Mar 14 '16 at 11:03
MattMatt
9111
9111
You could also use.transform
to reduce the amount of code... but that's a matter of personal preference.
– Pete
Mar 14 '16 at 15:22
As stated by Martijn in a previous comment, both.reduce
and.transform
update state outside the closure whereasmapValues
does not. So keep this in mind when using.reduce
.
– Pete
Mar 16 '16 at 21:37
@Pete Updating outside state is not an inherent property of.reduce
, but rather of the reducer itself. You can just as well use.reduce
without side-effects (see my short answer).
– hon2a
Mar 24 '16 at 10:42
@hon2a True... I one thing that bugs me about this particularreduce
solution, though, is that 'rel' and 'href' are hardcoded into the callback. It makes it less reusable. I tried to avoid that in the original solution. The maintenance cost of a one-liner to a five-liner is much more appealing to me. As for readability,reduce
is less fluent comparedkeyBy
andmapValues
to a non-programmer. Granted, all this is at the cost of performance and so it's not the end all of solutions. Thanks for updating to ES2015.
– Pete
Mar 24 '16 at 13:40
@Pete I'd argue that a simple use ofreduce
is actually better readable thankeyBy
+mapValues(propNameShortcut)
, asreduce
is a native (thus ubiquitous) method that doesn't require you to know the details of a specific (Lodash) API.
– hon2a
Apr 11 '16 at 10:39
|
show 2 more comments
You could also use.transform
to reduce the amount of code... but that's a matter of personal preference.
– Pete
Mar 14 '16 at 15:22
As stated by Martijn in a previous comment, both.reduce
and.transform
update state outside the closure whereasmapValues
does not. So keep this in mind when using.reduce
.
– Pete
Mar 16 '16 at 21:37
@Pete Updating outside state is not an inherent property of.reduce
, but rather of the reducer itself. You can just as well use.reduce
without side-effects (see my short answer).
– hon2a
Mar 24 '16 at 10:42
@hon2a True... I one thing that bugs me about this particularreduce
solution, though, is that 'rel' and 'href' are hardcoded into the callback. It makes it less reusable. I tried to avoid that in the original solution. The maintenance cost of a one-liner to a five-liner is much more appealing to me. As for readability,reduce
is less fluent comparedkeyBy
andmapValues
to a non-programmer. Granted, all this is at the cost of performance and so it's not the end all of solutions. Thanks for updating to ES2015.
– Pete
Mar 24 '16 at 13:40
@Pete I'd argue that a simple use ofreduce
is actually better readable thankeyBy
+mapValues(propNameShortcut)
, asreduce
is a native (thus ubiquitous) method that doesn't require you to know the details of a specific (Lodash) API.
– hon2a
Apr 11 '16 at 10:39
You could also use
.transform
to reduce the amount of code... but that's a matter of personal preference.– Pete
Mar 14 '16 at 15:22
You could also use
.transform
to reduce the amount of code... but that's a matter of personal preference.– Pete
Mar 14 '16 at 15:22
As stated by Martijn in a previous comment, both
.reduce
and .transform
update state outside the closure whereas mapValues
does not. So keep this in mind when using .reduce
.– Pete
Mar 16 '16 at 21:37
As stated by Martijn in a previous comment, both
.reduce
and .transform
update state outside the closure whereas mapValues
does not. So keep this in mind when using .reduce
.– Pete
Mar 16 '16 at 21:37
@Pete Updating outside state is not an inherent property of
.reduce
, but rather of the reducer itself. You can just as well use .reduce
without side-effects (see my short answer).– hon2a
Mar 24 '16 at 10:42
@Pete Updating outside state is not an inherent property of
.reduce
, but rather of the reducer itself. You can just as well use .reduce
without side-effects (see my short answer).– hon2a
Mar 24 '16 at 10:42
@hon2a True... I one thing that bugs me about this particular
reduce
solution, though, is that 'rel' and 'href' are hardcoded into the callback. It makes it less reusable. I tried to avoid that in the original solution. The maintenance cost of a one-liner to a five-liner is much more appealing to me. As for readability, reduce
is less fluent compared keyBy
and mapValues
to a non-programmer. Granted, all this is at the cost of performance and so it's not the end all of solutions. Thanks for updating to ES2015.– Pete
Mar 24 '16 at 13:40
@hon2a True... I one thing that bugs me about this particular
reduce
solution, though, is that 'rel' and 'href' are hardcoded into the callback. It makes it less reusable. I tried to avoid that in the original solution. The maintenance cost of a one-liner to a five-liner is much more appealing to me. As for readability, reduce
is less fluent compared keyBy
and mapValues
to a non-programmer. Granted, all this is at the cost of performance and so it's not the end all of solutions. Thanks for updating to ES2015.– Pete
Mar 24 '16 at 13:40
@Pete I'd argue that a simple use of
reduce
is actually better readable than keyBy
+ mapValues(propNameShortcut)
, as reduce
is a native (thus ubiquitous) method that doesn't require you to know the details of a specific (Lodash) API.– hon2a
Apr 11 '16 at 10:39
@Pete I'd argue that a simple use of
reduce
is actually better readable than keyBy
+ mapValues(propNameShortcut)
, as reduce
is a native (thus ubiquitous) method that doesn't require you to know the details of a specific (Lodash) API.– hon2a
Apr 11 '16 at 10:39
|
show 2 more comments
Since lodash 4, you can use _.fromPairs:
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
var hashmap = _.fromPairs(data.map(function(item) {
return [item.rel, item.href];
}));
1
Is there a way to parameterize 'rel' and 'href' outside of themap
method to make the solution more generic?
– Pete
May 25 '16 at 14:21
1
function makePair(keyProperty, valueProperty) { return function(item) { return [item[keyProperty], item[valueProperty]]; }; } var hashmap = _.fromPairs(data.map(makePair('rel', 'href')));
– Ricardo Stuven
Aug 28 '17 at 13:20
add a comment |
Since lodash 4, you can use _.fromPairs:
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
var hashmap = _.fromPairs(data.map(function(item) {
return [item.rel, item.href];
}));
1
Is there a way to parameterize 'rel' and 'href' outside of themap
method to make the solution more generic?
– Pete
May 25 '16 at 14:21
1
function makePair(keyProperty, valueProperty) { return function(item) { return [item[keyProperty], item[valueProperty]]; }; } var hashmap = _.fromPairs(data.map(makePair('rel', 'href')));
– Ricardo Stuven
Aug 28 '17 at 13:20
add a comment |
Since lodash 4, you can use _.fromPairs:
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
var hashmap = _.fromPairs(data.map(function(item) {
return [item.rel, item.href];
}));
Since lodash 4, you can use _.fromPairs:
var data = [
{ rel: 'link1', href: 'url1'},
{ rel: 'link2', href: 'url2'},
{ rel: 'link3', href: 'url3'},
{ rel: 'link4', href: 'url4'},
];
var hashmap = _.fromPairs(data.map(function(item) {
return [item.rel, item.href];
}));
edited Aug 3 '17 at 13:52
answered May 25 '16 at 14:05
Ricardo StuvenRicardo Stuven
16113
16113
1
Is there a way to parameterize 'rel' and 'href' outside of themap
method to make the solution more generic?
– Pete
May 25 '16 at 14:21
1
function makePair(keyProperty, valueProperty) { return function(item) { return [item[keyProperty], item[valueProperty]]; }; } var hashmap = _.fromPairs(data.map(makePair('rel', 'href')));
– Ricardo Stuven
Aug 28 '17 at 13:20
add a comment |
1
Is there a way to parameterize 'rel' and 'href' outside of themap
method to make the solution more generic?
– Pete
May 25 '16 at 14:21
1
function makePair(keyProperty, valueProperty) { return function(item) { return [item[keyProperty], item[valueProperty]]; }; } var hashmap = _.fromPairs(data.map(makePair('rel', 'href')));
– Ricardo Stuven
Aug 28 '17 at 13:20
1
1
Is there a way to parameterize 'rel' and 'href' outside of the
map
method to make the solution more generic?– Pete
May 25 '16 at 14:21
Is there a way to parameterize 'rel' and 'href' outside of the
map
method to make the solution more generic?– Pete
May 25 '16 at 14:21
1
1
function makePair(keyProperty, valueProperty) { return function(item) { return [item[keyProperty], item[valueProperty]]; }; } var hashmap = _.fromPairs(data.map(makePair('rel', 'href')));
– Ricardo Stuven
Aug 28 '17 at 13:20
function makePair(keyProperty, valueProperty) { return function(item) { return [item[keyProperty], item[valueProperty]]; }; } var hashmap = _.fromPairs(data.map(makePair('rel', 'href')));
– Ricardo Stuven
Aug 28 '17 at 13:20
add a comment |
With Lodash 4:
Using keyBy
and mapValues
_.mapValues(_.keyBy(data, 'rel'), v => v.href);
Or:
_.chain(data).keyBy('rel').mapValues(v => v.href).value();
add a comment |
With Lodash 4:
Using keyBy
and mapValues
_.mapValues(_.keyBy(data, 'rel'), v => v.href);
Or:
_.chain(data).keyBy('rel').mapValues(v => v.href).value();
add a comment |
With Lodash 4:
Using keyBy
and mapValues
_.mapValues(_.keyBy(data, 'rel'), v => v.href);
Or:
_.chain(data).keyBy('rel').mapValues(v => v.href).value();
With Lodash 4:
Using keyBy
and mapValues
_.mapValues(_.keyBy(data, 'rel'), v => v.href);
Or:
_.chain(data).keyBy('rel').mapValues(v => v.href).value();
edited Jan 19 '18 at 7:02
answered Mar 16 '17 at 18:18


rocketspacerrocketspacer
1213
1213
add a comment |
add a comment |
I see a lot of good answers already. I would just like to add one more answer to the list using Ramda's indexBy.
const data = [{
id: 1,
foo: 'bar'
},
{
id: 2,
baz: 'lorem'
}
];
const dataById = R.indexBy(R.prop('id'), data);
console.log(dataById)
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.25.0/ramda.min.js"></script>
I hope it helps someone else :)
This isn't really a review of the code provided, is it?
– Mast
Jan 15 '18 at 20:20
Sorry misunderstood the use of this website. More familiar with StackOverflow :)
– Abhishek Ghosh
Jan 16 '18 at 0:33
add a comment |
I see a lot of good answers already. I would just like to add one more answer to the list using Ramda's indexBy.
const data = [{
id: 1,
foo: 'bar'
},
{
id: 2,
baz: 'lorem'
}
];
const dataById = R.indexBy(R.prop('id'), data);
console.log(dataById)
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.25.0/ramda.min.js"></script>
I hope it helps someone else :)
This isn't really a review of the code provided, is it?
– Mast
Jan 15 '18 at 20:20
Sorry misunderstood the use of this website. More familiar with StackOverflow :)
– Abhishek Ghosh
Jan 16 '18 at 0:33
add a comment |
I see a lot of good answers already. I would just like to add one more answer to the list using Ramda's indexBy.
const data = [{
id: 1,
foo: 'bar'
},
{
id: 2,
baz: 'lorem'
}
];
const dataById = R.indexBy(R.prop('id'), data);
console.log(dataById)
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.25.0/ramda.min.js"></script>
I hope it helps someone else :)
I see a lot of good answers already. I would just like to add one more answer to the list using Ramda's indexBy.
const data = [{
id: 1,
foo: 'bar'
},
{
id: 2,
baz: 'lorem'
}
];
const dataById = R.indexBy(R.prop('id'), data);
console.log(dataById)
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.25.0/ramda.min.js"></script>
I hope it helps someone else :)
const data = [{
id: 1,
foo: 'bar'
},
{
id: 2,
baz: 'lorem'
}
];
const dataById = R.indexBy(R.prop('id'), data);
console.log(dataById)
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.25.0/ramda.min.js"></script>
const data = [{
id: 1,
foo: 'bar'
},
{
id: 2,
baz: 'lorem'
}
];
const dataById = R.indexBy(R.prop('id'), data);
console.log(dataById)
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.25.0/ramda.min.js"></script>
edited Jan 15 '18 at 20:31


Sᴀᴍ Onᴇᴌᴀ
9,03362157
9,03362157
answered Jan 15 '18 at 20:02


Abhishek GhoshAbhishek Ghosh
1012
1012
This isn't really a review of the code provided, is it?
– Mast
Jan 15 '18 at 20:20
Sorry misunderstood the use of this website. More familiar with StackOverflow :)
– Abhishek Ghosh
Jan 16 '18 at 0:33
add a comment |
This isn't really a review of the code provided, is it?
– Mast
Jan 15 '18 at 20:20
Sorry misunderstood the use of this website. More familiar with StackOverflow :)
– Abhishek Ghosh
Jan 16 '18 at 0:33
This isn't really a review of the code provided, is it?
– Mast
Jan 15 '18 at 20:20
This isn't really a review of the code provided, is it?
– Mast
Jan 15 '18 at 20:20
Sorry misunderstood the use of this website. More familiar with StackOverflow :)
– Abhishek Ghosh
Jan 16 '18 at 0:33
Sorry misunderstood the use of this website. More familiar with StackOverflow :)
– Abhishek Ghosh
Jan 16 '18 at 0:33
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f57614%2fconvert-object-array-to-hash-map-using-lodash%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Z,ARyt6ehHWyatuuVpMk7dF,uXMlDLU4I2,BBSg0x
I've compiled the most common ways to turn an object into an array in this lodash feature request (which currently needs upvotes!)
– BlueRaja - Danny Pflughoeft
Nov 17 '16 at 23:10