create a calculator with Powershell GUI
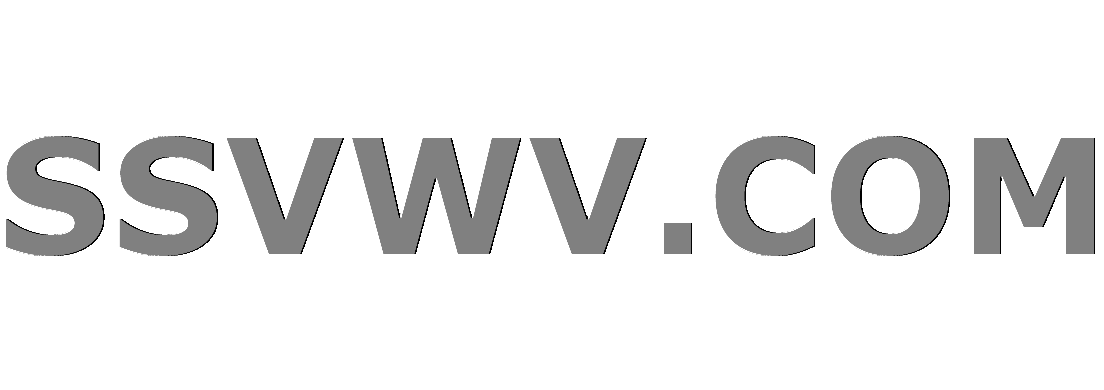
Multi tool use
So hello guys
Im trying to creat a calculator. So far I have the GUI finished. But i Need some help with the backgroundfunctions. Example: i type on the buttons 5+6. Now when i press "=" it should set the input of the TextBox into a variable and then calculate it out. Has somebody an idea how i can do that. (Im pretty knew to Powershell).
Thanks and sry for my bad Englisch.
Greetings
Sandro
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Drawing")
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
#Platfrom
$Taschenrechner = New-Object System.Windows.Forms.Form
$Taschenrechner.StartPosition = "CenterScreen"
$Taschenrechner.Size = New-Object System.Drawing.Size(470,400)
$Taschenrechner.Text = "Taschenrechner"
#Titel
$Titel = New-Object System.Windows.Forms.Label
$Titel.Location = New-Object System.Drawing.Size(70,10)
$Titel.Size = New-Object System.Drawing.Size(360,50)
$Font = New-Object System.Drawing.Font("Arial",30,[System.Drawing.FontStyle]::Regular)
$Titel.Font = $Font
$Titel.Text = "Taschenrechner"
$Titel.Name = "Taschenrechner"
$Taschenrechner.Controls.Add($Titel)
#Textfeld
$Textfeld= New-Object System.Windows.Forms.TextBox
$Textfeld.Location = New-Object System.Drawing.Size(25,100)
$Textfeld.Size = New-Object System.Drawing.Size(100,100)
$Textfeld.Width = (400)
$Taschenrechner.Controls.Add($Textfeld)
#1
$button1 = New-Object System.Windows.Forms.Button
$button1.Location = New-Object System.Drawing.Size(25,120)
$button1.Size = New-Object System.Drawing.Size(100,50)
$button1.Add_Click({$Textfeld.Text+='1'})
$button1.Text = "1"
$button1.Name = "1"
$Taschenrechner.Controls.Add($button1)
#2
$button2 = New-Object System.Windows.Forms.Button
$button2.Location = New-Object System.Drawing.Size(125,120)
$button2.Size = New-Object System.Drawing.Size(100,50)
$button2.Add_Click({$Textfeld.Text+='2'})
$button2.Text = "2"
$button2.Name = "2"
$Taschenrechner.Controls.Add($button2)
#3
$button3 = New-Object System.Windows.Forms.Button
$button3.Location = New-Object System.Drawing.Size(225,120)
$button3.Size = New-Object System.Drawing.Size(100,50)
$button3.Add_Click({$Textfeld.Text+='3'})
$button3.Text = "3"
$button3.Name = "3"
$Taschenrechner.Controls.Add($button3)
#4
$button4 = New-Object System.Windows.Forms.Button
$button4.Location = New-Object System.Drawing.Size(25,170)
$button4.Size = New-Object System.Drawing.Size(100,50)
$button4.Add_Click({$Textfeld.Text+='4'})
$button4.Text = "4"
$button4.Name = "4"
$Taschenrechner.Controls.Add($button4)
#5
$button5 = New-Object System.Windows.Forms.Button
$button5.Location = New-Object System.Drawing.Size(125,170)
$button5.Size = New-Object System.Drawing.Size(100,50)
$button5.Add_Click({$Textfeld.Text+='5'})
$button5.Text = "5"
$button5.Name = "5"
$Taschenrechner.Controls.Add($button5)
#6
$button6 = New-Object System.Windows.Forms.Button
$button6.Location = New-Object System.Drawing.Size(225,170)
$button6.Size = New-Object System.Drawing.Size(100,50)
$button6.Add_Click({$Textfeld.Text+='6'})
$button6.Text = "6"
$button6.Name = "6"
$Taschenrechner.Controls.Add($button6)
#7
$button7 = New-Object System.Windows.Forms.Button
$button7.Location = New-Object System.Drawing.Size(25,220)
$button7.Size = New-Object System.Drawing.Size(100,50)
$button7.Add_Click({$Textfeld.Text+='7'})
$button7.Text = "7"
$button7.Name = "7"
$Taschenrechner.Controls.Add($button7)
#8
$button8 = New-Object System.Windows.Forms.Button
$button8.Location = New-Object System.Drawing.Size(125,220)
$button8.Size = New-Object System.Drawing.Size(100,50)
$button8.Add_Click({$Textfeld.Text+='8'})
$button8.Text = "8"
$button8.Name = "8"
$Taschenrechner.Controls.Add($button8)
#9
$button9 = New-Object System.Windows.Forms.Button
$button9.Location = New-Object System.Drawing.Size(225,220)
$button9.Size = New-Object System.Drawing.Size(100,50)
$button9.Add_Click({$Textfeld.Text+='9'})
$button9.Text = "9"
$button9.Name = "9"
$Taschenrechner.Controls.Add($button9)
#0
$button0 = New-Object System.Windows.Forms.Button
$button0.Location = New-Object System.Drawing.Size(25,270)
$button0.Size = New-Object System.Drawing.Size(100,50)
$button0.Add_Click({$Textfeld.Text+='0'})
$button0.Text = "0"
$button0.Name = "0"
$Taschenrechner.Controls.Add($button0)
#Dezimalstelle
$Punkt = New-Object System.Windows.Forms.Button
$Punkt.Location = New-Object System.Drawing.Size(125,270)
$Punkt.Size = New-Object System.Drawing.Size(100,50)
$Punkt.Add_Click({$Textfeld.Text+='.'})
$Punkt.Text = "."
$Punkt.Name = "."
$Taschenrechner.Controls.Add($Punkt)
#Gleich
$gleich = New-Object System.Windows.Forms.Button
$gleich.Location = New-Object System.Drawing.Size(225,270)
$gleich.Size = New-Object System.Drawing.Size(100,50)
$gleich.Text = "="
$gleich.Name = "="
$Taschenrechner.Controls.Add($gleich)
#Durch
$durch = New-Object System.Windows.Forms.Button
$durch.Location = New-Object System.Drawing.Size(325,120)
$durch.Size = New-Object System.Drawing.Size(100,50)
$durch.Text = "/"
$durch.Name = "/"
$Taschenrechner.Controls.Add($durch)
#Mal
$mal = New-Object System.Windows.Forms.Button
$mal.Location = New-Object System.Drawing.Size(325,170)
$mal.Size = New-Object System.Drawing.Size(100,50)
$mal.Text = "x"
$mal.Name = "x"
$Taschenrechner.Controls.Add($mal)
#Minus
$minus = New-Object System.Windows.Forms.Button
$minus.Location = New-Object System.Drawing.Size(325,220)
$minus.Size = New-Object System.Drawing.Size(100,50)
$minus.Text = "-"
$minus.Name = "-"
$Taschenrechner.Controls.Add($minus)
#Plus
$plus = New-Object System.Windows.Forms.Button
$plus.Location = New-Object System.Drawing.Size(325,270)
$plus.Size = New-Object System.Drawing.Size(100,50)
$plus.Text = "+"
$plus.Name = "+"
$Taschenrechner.Controls.Add($plus)
[void] $Taschenrechner.ShowDialog()
powershell gui powershell-ise
add a comment |
So hello guys
Im trying to creat a calculator. So far I have the GUI finished. But i Need some help with the backgroundfunctions. Example: i type on the buttons 5+6. Now when i press "=" it should set the input of the TextBox into a variable and then calculate it out. Has somebody an idea how i can do that. (Im pretty knew to Powershell).
Thanks and sry for my bad Englisch.
Greetings
Sandro
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Drawing")
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
#Platfrom
$Taschenrechner = New-Object System.Windows.Forms.Form
$Taschenrechner.StartPosition = "CenterScreen"
$Taschenrechner.Size = New-Object System.Drawing.Size(470,400)
$Taschenrechner.Text = "Taschenrechner"
#Titel
$Titel = New-Object System.Windows.Forms.Label
$Titel.Location = New-Object System.Drawing.Size(70,10)
$Titel.Size = New-Object System.Drawing.Size(360,50)
$Font = New-Object System.Drawing.Font("Arial",30,[System.Drawing.FontStyle]::Regular)
$Titel.Font = $Font
$Titel.Text = "Taschenrechner"
$Titel.Name = "Taschenrechner"
$Taschenrechner.Controls.Add($Titel)
#Textfeld
$Textfeld= New-Object System.Windows.Forms.TextBox
$Textfeld.Location = New-Object System.Drawing.Size(25,100)
$Textfeld.Size = New-Object System.Drawing.Size(100,100)
$Textfeld.Width = (400)
$Taschenrechner.Controls.Add($Textfeld)
#1
$button1 = New-Object System.Windows.Forms.Button
$button1.Location = New-Object System.Drawing.Size(25,120)
$button1.Size = New-Object System.Drawing.Size(100,50)
$button1.Add_Click({$Textfeld.Text+='1'})
$button1.Text = "1"
$button1.Name = "1"
$Taschenrechner.Controls.Add($button1)
#2
$button2 = New-Object System.Windows.Forms.Button
$button2.Location = New-Object System.Drawing.Size(125,120)
$button2.Size = New-Object System.Drawing.Size(100,50)
$button2.Add_Click({$Textfeld.Text+='2'})
$button2.Text = "2"
$button2.Name = "2"
$Taschenrechner.Controls.Add($button2)
#3
$button3 = New-Object System.Windows.Forms.Button
$button3.Location = New-Object System.Drawing.Size(225,120)
$button3.Size = New-Object System.Drawing.Size(100,50)
$button3.Add_Click({$Textfeld.Text+='3'})
$button3.Text = "3"
$button3.Name = "3"
$Taschenrechner.Controls.Add($button3)
#4
$button4 = New-Object System.Windows.Forms.Button
$button4.Location = New-Object System.Drawing.Size(25,170)
$button4.Size = New-Object System.Drawing.Size(100,50)
$button4.Add_Click({$Textfeld.Text+='4'})
$button4.Text = "4"
$button4.Name = "4"
$Taschenrechner.Controls.Add($button4)
#5
$button5 = New-Object System.Windows.Forms.Button
$button5.Location = New-Object System.Drawing.Size(125,170)
$button5.Size = New-Object System.Drawing.Size(100,50)
$button5.Add_Click({$Textfeld.Text+='5'})
$button5.Text = "5"
$button5.Name = "5"
$Taschenrechner.Controls.Add($button5)
#6
$button6 = New-Object System.Windows.Forms.Button
$button6.Location = New-Object System.Drawing.Size(225,170)
$button6.Size = New-Object System.Drawing.Size(100,50)
$button6.Add_Click({$Textfeld.Text+='6'})
$button6.Text = "6"
$button6.Name = "6"
$Taschenrechner.Controls.Add($button6)
#7
$button7 = New-Object System.Windows.Forms.Button
$button7.Location = New-Object System.Drawing.Size(25,220)
$button7.Size = New-Object System.Drawing.Size(100,50)
$button7.Add_Click({$Textfeld.Text+='7'})
$button7.Text = "7"
$button7.Name = "7"
$Taschenrechner.Controls.Add($button7)
#8
$button8 = New-Object System.Windows.Forms.Button
$button8.Location = New-Object System.Drawing.Size(125,220)
$button8.Size = New-Object System.Drawing.Size(100,50)
$button8.Add_Click({$Textfeld.Text+='8'})
$button8.Text = "8"
$button8.Name = "8"
$Taschenrechner.Controls.Add($button8)
#9
$button9 = New-Object System.Windows.Forms.Button
$button9.Location = New-Object System.Drawing.Size(225,220)
$button9.Size = New-Object System.Drawing.Size(100,50)
$button9.Add_Click({$Textfeld.Text+='9'})
$button9.Text = "9"
$button9.Name = "9"
$Taschenrechner.Controls.Add($button9)
#0
$button0 = New-Object System.Windows.Forms.Button
$button0.Location = New-Object System.Drawing.Size(25,270)
$button0.Size = New-Object System.Drawing.Size(100,50)
$button0.Add_Click({$Textfeld.Text+='0'})
$button0.Text = "0"
$button0.Name = "0"
$Taschenrechner.Controls.Add($button0)
#Dezimalstelle
$Punkt = New-Object System.Windows.Forms.Button
$Punkt.Location = New-Object System.Drawing.Size(125,270)
$Punkt.Size = New-Object System.Drawing.Size(100,50)
$Punkt.Add_Click({$Textfeld.Text+='.'})
$Punkt.Text = "."
$Punkt.Name = "."
$Taschenrechner.Controls.Add($Punkt)
#Gleich
$gleich = New-Object System.Windows.Forms.Button
$gleich.Location = New-Object System.Drawing.Size(225,270)
$gleich.Size = New-Object System.Drawing.Size(100,50)
$gleich.Text = "="
$gleich.Name = "="
$Taschenrechner.Controls.Add($gleich)
#Durch
$durch = New-Object System.Windows.Forms.Button
$durch.Location = New-Object System.Drawing.Size(325,120)
$durch.Size = New-Object System.Drawing.Size(100,50)
$durch.Text = "/"
$durch.Name = "/"
$Taschenrechner.Controls.Add($durch)
#Mal
$mal = New-Object System.Windows.Forms.Button
$mal.Location = New-Object System.Drawing.Size(325,170)
$mal.Size = New-Object System.Drawing.Size(100,50)
$mal.Text = "x"
$mal.Name = "x"
$Taschenrechner.Controls.Add($mal)
#Minus
$minus = New-Object System.Windows.Forms.Button
$minus.Location = New-Object System.Drawing.Size(325,220)
$minus.Size = New-Object System.Drawing.Size(100,50)
$minus.Text = "-"
$minus.Name = "-"
$Taschenrechner.Controls.Add($minus)
#Plus
$plus = New-Object System.Windows.Forms.Button
$plus.Location = New-Object System.Drawing.Size(325,270)
$plus.Size = New-Object System.Drawing.Size(100,50)
$plus.Text = "+"
$plus.Name = "+"
$Taschenrechner.Controls.Add($plus)
[void] $Taschenrechner.ShowDialog()
powershell gui powershell-ise
1
If you're pulling those stunts with PowerShell just go ahead and write a "real" C# Program. it's going to be less of a headache and using Visual Studio would mean you would have a lot of GUI help for a lot of things (IntelliSense etc.). What you would need to do is bind to the event for the buttons and let the function you bind to the event change the text for the input.
– Seth
Jan 21 '17 at 15:24
add a comment |
So hello guys
Im trying to creat a calculator. So far I have the GUI finished. But i Need some help with the backgroundfunctions. Example: i type on the buttons 5+6. Now when i press "=" it should set the input of the TextBox into a variable and then calculate it out. Has somebody an idea how i can do that. (Im pretty knew to Powershell).
Thanks and sry for my bad Englisch.
Greetings
Sandro
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Drawing")
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
#Platfrom
$Taschenrechner = New-Object System.Windows.Forms.Form
$Taschenrechner.StartPosition = "CenterScreen"
$Taschenrechner.Size = New-Object System.Drawing.Size(470,400)
$Taschenrechner.Text = "Taschenrechner"
#Titel
$Titel = New-Object System.Windows.Forms.Label
$Titel.Location = New-Object System.Drawing.Size(70,10)
$Titel.Size = New-Object System.Drawing.Size(360,50)
$Font = New-Object System.Drawing.Font("Arial",30,[System.Drawing.FontStyle]::Regular)
$Titel.Font = $Font
$Titel.Text = "Taschenrechner"
$Titel.Name = "Taschenrechner"
$Taschenrechner.Controls.Add($Titel)
#Textfeld
$Textfeld= New-Object System.Windows.Forms.TextBox
$Textfeld.Location = New-Object System.Drawing.Size(25,100)
$Textfeld.Size = New-Object System.Drawing.Size(100,100)
$Textfeld.Width = (400)
$Taschenrechner.Controls.Add($Textfeld)
#1
$button1 = New-Object System.Windows.Forms.Button
$button1.Location = New-Object System.Drawing.Size(25,120)
$button1.Size = New-Object System.Drawing.Size(100,50)
$button1.Add_Click({$Textfeld.Text+='1'})
$button1.Text = "1"
$button1.Name = "1"
$Taschenrechner.Controls.Add($button1)
#2
$button2 = New-Object System.Windows.Forms.Button
$button2.Location = New-Object System.Drawing.Size(125,120)
$button2.Size = New-Object System.Drawing.Size(100,50)
$button2.Add_Click({$Textfeld.Text+='2'})
$button2.Text = "2"
$button2.Name = "2"
$Taschenrechner.Controls.Add($button2)
#3
$button3 = New-Object System.Windows.Forms.Button
$button3.Location = New-Object System.Drawing.Size(225,120)
$button3.Size = New-Object System.Drawing.Size(100,50)
$button3.Add_Click({$Textfeld.Text+='3'})
$button3.Text = "3"
$button3.Name = "3"
$Taschenrechner.Controls.Add($button3)
#4
$button4 = New-Object System.Windows.Forms.Button
$button4.Location = New-Object System.Drawing.Size(25,170)
$button4.Size = New-Object System.Drawing.Size(100,50)
$button4.Add_Click({$Textfeld.Text+='4'})
$button4.Text = "4"
$button4.Name = "4"
$Taschenrechner.Controls.Add($button4)
#5
$button5 = New-Object System.Windows.Forms.Button
$button5.Location = New-Object System.Drawing.Size(125,170)
$button5.Size = New-Object System.Drawing.Size(100,50)
$button5.Add_Click({$Textfeld.Text+='5'})
$button5.Text = "5"
$button5.Name = "5"
$Taschenrechner.Controls.Add($button5)
#6
$button6 = New-Object System.Windows.Forms.Button
$button6.Location = New-Object System.Drawing.Size(225,170)
$button6.Size = New-Object System.Drawing.Size(100,50)
$button6.Add_Click({$Textfeld.Text+='6'})
$button6.Text = "6"
$button6.Name = "6"
$Taschenrechner.Controls.Add($button6)
#7
$button7 = New-Object System.Windows.Forms.Button
$button7.Location = New-Object System.Drawing.Size(25,220)
$button7.Size = New-Object System.Drawing.Size(100,50)
$button7.Add_Click({$Textfeld.Text+='7'})
$button7.Text = "7"
$button7.Name = "7"
$Taschenrechner.Controls.Add($button7)
#8
$button8 = New-Object System.Windows.Forms.Button
$button8.Location = New-Object System.Drawing.Size(125,220)
$button8.Size = New-Object System.Drawing.Size(100,50)
$button8.Add_Click({$Textfeld.Text+='8'})
$button8.Text = "8"
$button8.Name = "8"
$Taschenrechner.Controls.Add($button8)
#9
$button9 = New-Object System.Windows.Forms.Button
$button9.Location = New-Object System.Drawing.Size(225,220)
$button9.Size = New-Object System.Drawing.Size(100,50)
$button9.Add_Click({$Textfeld.Text+='9'})
$button9.Text = "9"
$button9.Name = "9"
$Taschenrechner.Controls.Add($button9)
#0
$button0 = New-Object System.Windows.Forms.Button
$button0.Location = New-Object System.Drawing.Size(25,270)
$button0.Size = New-Object System.Drawing.Size(100,50)
$button0.Add_Click({$Textfeld.Text+='0'})
$button0.Text = "0"
$button0.Name = "0"
$Taschenrechner.Controls.Add($button0)
#Dezimalstelle
$Punkt = New-Object System.Windows.Forms.Button
$Punkt.Location = New-Object System.Drawing.Size(125,270)
$Punkt.Size = New-Object System.Drawing.Size(100,50)
$Punkt.Add_Click({$Textfeld.Text+='.'})
$Punkt.Text = "."
$Punkt.Name = "."
$Taschenrechner.Controls.Add($Punkt)
#Gleich
$gleich = New-Object System.Windows.Forms.Button
$gleich.Location = New-Object System.Drawing.Size(225,270)
$gleich.Size = New-Object System.Drawing.Size(100,50)
$gleich.Text = "="
$gleich.Name = "="
$Taschenrechner.Controls.Add($gleich)
#Durch
$durch = New-Object System.Windows.Forms.Button
$durch.Location = New-Object System.Drawing.Size(325,120)
$durch.Size = New-Object System.Drawing.Size(100,50)
$durch.Text = "/"
$durch.Name = "/"
$Taschenrechner.Controls.Add($durch)
#Mal
$mal = New-Object System.Windows.Forms.Button
$mal.Location = New-Object System.Drawing.Size(325,170)
$mal.Size = New-Object System.Drawing.Size(100,50)
$mal.Text = "x"
$mal.Name = "x"
$Taschenrechner.Controls.Add($mal)
#Minus
$minus = New-Object System.Windows.Forms.Button
$minus.Location = New-Object System.Drawing.Size(325,220)
$minus.Size = New-Object System.Drawing.Size(100,50)
$minus.Text = "-"
$minus.Name = "-"
$Taschenrechner.Controls.Add($minus)
#Plus
$plus = New-Object System.Windows.Forms.Button
$plus.Location = New-Object System.Drawing.Size(325,270)
$plus.Size = New-Object System.Drawing.Size(100,50)
$plus.Text = "+"
$plus.Name = "+"
$Taschenrechner.Controls.Add($plus)
[void] $Taschenrechner.ShowDialog()
powershell gui powershell-ise
So hello guys
Im trying to creat a calculator. So far I have the GUI finished. But i Need some help with the backgroundfunctions. Example: i type on the buttons 5+6. Now when i press "=" it should set the input of the TextBox into a variable and then calculate it out. Has somebody an idea how i can do that. (Im pretty knew to Powershell).
Thanks and sry for my bad Englisch.
Greetings
Sandro
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Drawing")
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
#Platfrom
$Taschenrechner = New-Object System.Windows.Forms.Form
$Taschenrechner.StartPosition = "CenterScreen"
$Taschenrechner.Size = New-Object System.Drawing.Size(470,400)
$Taschenrechner.Text = "Taschenrechner"
#Titel
$Titel = New-Object System.Windows.Forms.Label
$Titel.Location = New-Object System.Drawing.Size(70,10)
$Titel.Size = New-Object System.Drawing.Size(360,50)
$Font = New-Object System.Drawing.Font("Arial",30,[System.Drawing.FontStyle]::Regular)
$Titel.Font = $Font
$Titel.Text = "Taschenrechner"
$Titel.Name = "Taschenrechner"
$Taschenrechner.Controls.Add($Titel)
#Textfeld
$Textfeld= New-Object System.Windows.Forms.TextBox
$Textfeld.Location = New-Object System.Drawing.Size(25,100)
$Textfeld.Size = New-Object System.Drawing.Size(100,100)
$Textfeld.Width = (400)
$Taschenrechner.Controls.Add($Textfeld)
#1
$button1 = New-Object System.Windows.Forms.Button
$button1.Location = New-Object System.Drawing.Size(25,120)
$button1.Size = New-Object System.Drawing.Size(100,50)
$button1.Add_Click({$Textfeld.Text+='1'})
$button1.Text = "1"
$button1.Name = "1"
$Taschenrechner.Controls.Add($button1)
#2
$button2 = New-Object System.Windows.Forms.Button
$button2.Location = New-Object System.Drawing.Size(125,120)
$button2.Size = New-Object System.Drawing.Size(100,50)
$button2.Add_Click({$Textfeld.Text+='2'})
$button2.Text = "2"
$button2.Name = "2"
$Taschenrechner.Controls.Add($button2)
#3
$button3 = New-Object System.Windows.Forms.Button
$button3.Location = New-Object System.Drawing.Size(225,120)
$button3.Size = New-Object System.Drawing.Size(100,50)
$button3.Add_Click({$Textfeld.Text+='3'})
$button3.Text = "3"
$button3.Name = "3"
$Taschenrechner.Controls.Add($button3)
#4
$button4 = New-Object System.Windows.Forms.Button
$button4.Location = New-Object System.Drawing.Size(25,170)
$button4.Size = New-Object System.Drawing.Size(100,50)
$button4.Add_Click({$Textfeld.Text+='4'})
$button4.Text = "4"
$button4.Name = "4"
$Taschenrechner.Controls.Add($button4)
#5
$button5 = New-Object System.Windows.Forms.Button
$button5.Location = New-Object System.Drawing.Size(125,170)
$button5.Size = New-Object System.Drawing.Size(100,50)
$button5.Add_Click({$Textfeld.Text+='5'})
$button5.Text = "5"
$button5.Name = "5"
$Taschenrechner.Controls.Add($button5)
#6
$button6 = New-Object System.Windows.Forms.Button
$button6.Location = New-Object System.Drawing.Size(225,170)
$button6.Size = New-Object System.Drawing.Size(100,50)
$button6.Add_Click({$Textfeld.Text+='6'})
$button6.Text = "6"
$button6.Name = "6"
$Taschenrechner.Controls.Add($button6)
#7
$button7 = New-Object System.Windows.Forms.Button
$button7.Location = New-Object System.Drawing.Size(25,220)
$button7.Size = New-Object System.Drawing.Size(100,50)
$button7.Add_Click({$Textfeld.Text+='7'})
$button7.Text = "7"
$button7.Name = "7"
$Taschenrechner.Controls.Add($button7)
#8
$button8 = New-Object System.Windows.Forms.Button
$button8.Location = New-Object System.Drawing.Size(125,220)
$button8.Size = New-Object System.Drawing.Size(100,50)
$button8.Add_Click({$Textfeld.Text+='8'})
$button8.Text = "8"
$button8.Name = "8"
$Taschenrechner.Controls.Add($button8)
#9
$button9 = New-Object System.Windows.Forms.Button
$button9.Location = New-Object System.Drawing.Size(225,220)
$button9.Size = New-Object System.Drawing.Size(100,50)
$button9.Add_Click({$Textfeld.Text+='9'})
$button9.Text = "9"
$button9.Name = "9"
$Taschenrechner.Controls.Add($button9)
#0
$button0 = New-Object System.Windows.Forms.Button
$button0.Location = New-Object System.Drawing.Size(25,270)
$button0.Size = New-Object System.Drawing.Size(100,50)
$button0.Add_Click({$Textfeld.Text+='0'})
$button0.Text = "0"
$button0.Name = "0"
$Taschenrechner.Controls.Add($button0)
#Dezimalstelle
$Punkt = New-Object System.Windows.Forms.Button
$Punkt.Location = New-Object System.Drawing.Size(125,270)
$Punkt.Size = New-Object System.Drawing.Size(100,50)
$Punkt.Add_Click({$Textfeld.Text+='.'})
$Punkt.Text = "."
$Punkt.Name = "."
$Taschenrechner.Controls.Add($Punkt)
#Gleich
$gleich = New-Object System.Windows.Forms.Button
$gleich.Location = New-Object System.Drawing.Size(225,270)
$gleich.Size = New-Object System.Drawing.Size(100,50)
$gleich.Text = "="
$gleich.Name = "="
$Taschenrechner.Controls.Add($gleich)
#Durch
$durch = New-Object System.Windows.Forms.Button
$durch.Location = New-Object System.Drawing.Size(325,120)
$durch.Size = New-Object System.Drawing.Size(100,50)
$durch.Text = "/"
$durch.Name = "/"
$Taschenrechner.Controls.Add($durch)
#Mal
$mal = New-Object System.Windows.Forms.Button
$mal.Location = New-Object System.Drawing.Size(325,170)
$mal.Size = New-Object System.Drawing.Size(100,50)
$mal.Text = "x"
$mal.Name = "x"
$Taschenrechner.Controls.Add($mal)
#Minus
$minus = New-Object System.Windows.Forms.Button
$minus.Location = New-Object System.Drawing.Size(325,220)
$minus.Size = New-Object System.Drawing.Size(100,50)
$minus.Text = "-"
$minus.Name = "-"
$Taschenrechner.Controls.Add($minus)
#Plus
$plus = New-Object System.Windows.Forms.Button
$plus.Location = New-Object System.Drawing.Size(325,270)
$plus.Size = New-Object System.Drawing.Size(100,50)
$plus.Text = "+"
$plus.Name = "+"
$Taschenrechner.Controls.Add($plus)
[void] $Taschenrechner.ShowDialog()
powershell gui powershell-ise
powershell gui powershell-ise
asked Jan 21 '17 at 15:20
Sandro21Sandro21
312
312
1
If you're pulling those stunts with PowerShell just go ahead and write a "real" C# Program. it's going to be less of a headache and using Visual Studio would mean you would have a lot of GUI help for a lot of things (IntelliSense etc.). What you would need to do is bind to the event for the buttons and let the function you bind to the event change the text for the input.
– Seth
Jan 21 '17 at 15:24
add a comment |
1
If you're pulling those stunts with PowerShell just go ahead and write a "real" C# Program. it's going to be less of a headache and using Visual Studio would mean you would have a lot of GUI help for a lot of things (IntelliSense etc.). What you would need to do is bind to the event for the buttons and let the function you bind to the event change the text for the input.
– Seth
Jan 21 '17 at 15:24
1
1
If you're pulling those stunts with PowerShell just go ahead and write a "real" C# Program. it's going to be less of a headache and using Visual Studio would mean you would have a lot of GUI help for a lot of things (IntelliSense etc.). What you would need to do is bind to the event for the buttons and let the function you bind to the event change the text for the input.
– Seth
Jan 21 '17 at 15:24
If you're pulling those stunts with PowerShell just go ahead and write a "real" C# Program. it's going to be less of a headache and using Visual Studio would mean you would have a lot of GUI help for a lot of things (IntelliSense etc.). What you would need to do is bind to the event for the buttons and let the function you bind to the event change the text for the input.
– Seth
Jan 21 '17 at 15:24
add a comment |
1 Answer
1
active
oldest
votes
The following improvement of my previous answer to your almost the same question could help however such patchwork would conduce you to perdition (as well as me). Good luck!
Remove-Variable script:Part* -ErrorAction SilentlyContinue
$script:PartialResult = ''
$script:PartOperation = ''
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Drawing")
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
#Platfrom
### unchanged code here (cca 110 lines)
#Dezimalstelle
$Punkt = New-Object System.Windows.Forms.Button
$Punkt.Location = New-Object System.Drawing.Size(225,270)
$Punkt.Size = New-Object System.Drawing.Size(100,50)
$Punkt.Add_Click({$Textfeld.Text+='.'})
$Punkt.Text = "."
$Punkt.Name = "."
$Taschenrechner.Controls.Add($Punkt)
#Gleich
$gleich = New-Object System.Windows.Forms.Button
$gleich.Location = New-Object System.Drawing.Size(325,270)
$gleich.Size = New-Object System.Drawing.Size(100,50)
$gleich.Text = "="
$gleich.Name = "="
$Taschenrechner.Controls.Add($gleich)
#Durch
$durch = New-Object System.Windows.Forms.Button
$durch.Location = New-Object System.Drawing.Size(425,120)
$durch.Size = New-Object System.Drawing.Size(100,50)
$durch.Text = "/"
$durch.Name = "/"
$Taschenrechner.Controls.Add($durch)
#Mal
$mal = New-Object System.Windows.Forms.Button
$mal.Location = New-Object System.Drawing.Size(425,170)
$mal.Size = New-Object System.Drawing.Size(100,50)
$mal.Text = "×"
$mal.Name = "*"
$Taschenrechner.Controls.Add($mal)
#Minus
$minus = New-Object System.Windows.Forms.Button
$minus.Location = New-Object System.Drawing.Size(425,220)
$minus.Size = New-Object System.Drawing.Size(100,50)
$minus.Text = "-"
$minus.Name = "-"
$Taschenrechner.Controls.Add($minus)
#Plus
$plus = New-Object System.Windows.Forms.Button
$plus.Location = New-Object System.Drawing.Size(425,270)
$plus.Size = New-Object System.Drawing.Size(100,50)
$plus.Text = "+"
$plus.Name = "+"
$Taschenrechner.Controls.Add($plus)
Function Operation {
param([string] $Ope)
### you need to show partial result an operation!!!
if ( $Textfeld.Text -ne '' ) {
if ($script:PartialResult -ne '' ) {
$aux = [string] $script:PartialResult + $script:PartOperation + $script:Textfeld.Text
$script:PartialResult = [string] $( Invoke-Expression $aux )
} else {
$script:PartialResult = $Textfeld.Text
}
if ( $Ope -eq '=' ) {
$Textfeld.Text = '' + [string] $script:PartialResult
$script:PartialResult = ''
$script:PartOperation = ''
} else {
$Textfeld.Text = ''
$script:PartOperation = $Ope
}
}
}
$gleich.Add_Click( { Operation '=' } )
$mal. Add_Click( { Operation '*' } ) # Name = "×"
$plus. Add_Click( { Operation '+' } ) # Name = "+"
$minus. Add_Click( { Operation '-' } ) # Name = "-"
$durch. Add_Click( { Operation '/' } ) # Name = "/"
[void] $Taschenrechner.ShowDialog()
Hello JosefZ thanks for your answer. Could you explain me what the Funtion Operation exactly is doing?
– Sandro21
Jan 22 '17 at 11:43
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "3"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsuperuser.com%2fquestions%2f1170043%2fcreate-a-calculator-with-powershell-gui%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The following improvement of my previous answer to your almost the same question could help however such patchwork would conduce you to perdition (as well as me). Good luck!
Remove-Variable script:Part* -ErrorAction SilentlyContinue
$script:PartialResult = ''
$script:PartOperation = ''
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Drawing")
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
#Platfrom
### unchanged code here (cca 110 lines)
#Dezimalstelle
$Punkt = New-Object System.Windows.Forms.Button
$Punkt.Location = New-Object System.Drawing.Size(225,270)
$Punkt.Size = New-Object System.Drawing.Size(100,50)
$Punkt.Add_Click({$Textfeld.Text+='.'})
$Punkt.Text = "."
$Punkt.Name = "."
$Taschenrechner.Controls.Add($Punkt)
#Gleich
$gleich = New-Object System.Windows.Forms.Button
$gleich.Location = New-Object System.Drawing.Size(325,270)
$gleich.Size = New-Object System.Drawing.Size(100,50)
$gleich.Text = "="
$gleich.Name = "="
$Taschenrechner.Controls.Add($gleich)
#Durch
$durch = New-Object System.Windows.Forms.Button
$durch.Location = New-Object System.Drawing.Size(425,120)
$durch.Size = New-Object System.Drawing.Size(100,50)
$durch.Text = "/"
$durch.Name = "/"
$Taschenrechner.Controls.Add($durch)
#Mal
$mal = New-Object System.Windows.Forms.Button
$mal.Location = New-Object System.Drawing.Size(425,170)
$mal.Size = New-Object System.Drawing.Size(100,50)
$mal.Text = "×"
$mal.Name = "*"
$Taschenrechner.Controls.Add($mal)
#Minus
$minus = New-Object System.Windows.Forms.Button
$minus.Location = New-Object System.Drawing.Size(425,220)
$minus.Size = New-Object System.Drawing.Size(100,50)
$minus.Text = "-"
$minus.Name = "-"
$Taschenrechner.Controls.Add($minus)
#Plus
$plus = New-Object System.Windows.Forms.Button
$plus.Location = New-Object System.Drawing.Size(425,270)
$plus.Size = New-Object System.Drawing.Size(100,50)
$plus.Text = "+"
$plus.Name = "+"
$Taschenrechner.Controls.Add($plus)
Function Operation {
param([string] $Ope)
### you need to show partial result an operation!!!
if ( $Textfeld.Text -ne '' ) {
if ($script:PartialResult -ne '' ) {
$aux = [string] $script:PartialResult + $script:PartOperation + $script:Textfeld.Text
$script:PartialResult = [string] $( Invoke-Expression $aux )
} else {
$script:PartialResult = $Textfeld.Text
}
if ( $Ope -eq '=' ) {
$Textfeld.Text = '' + [string] $script:PartialResult
$script:PartialResult = ''
$script:PartOperation = ''
} else {
$Textfeld.Text = ''
$script:PartOperation = $Ope
}
}
}
$gleich.Add_Click( { Operation '=' } )
$mal. Add_Click( { Operation '*' } ) # Name = "×"
$plus. Add_Click( { Operation '+' } ) # Name = "+"
$minus. Add_Click( { Operation '-' } ) # Name = "-"
$durch. Add_Click( { Operation '/' } ) # Name = "/"
[void] $Taschenrechner.ShowDialog()
Hello JosefZ thanks for your answer. Could you explain me what the Funtion Operation exactly is doing?
– Sandro21
Jan 22 '17 at 11:43
add a comment |
The following improvement of my previous answer to your almost the same question could help however such patchwork would conduce you to perdition (as well as me). Good luck!
Remove-Variable script:Part* -ErrorAction SilentlyContinue
$script:PartialResult = ''
$script:PartOperation = ''
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Drawing")
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
#Platfrom
### unchanged code here (cca 110 lines)
#Dezimalstelle
$Punkt = New-Object System.Windows.Forms.Button
$Punkt.Location = New-Object System.Drawing.Size(225,270)
$Punkt.Size = New-Object System.Drawing.Size(100,50)
$Punkt.Add_Click({$Textfeld.Text+='.'})
$Punkt.Text = "."
$Punkt.Name = "."
$Taschenrechner.Controls.Add($Punkt)
#Gleich
$gleich = New-Object System.Windows.Forms.Button
$gleich.Location = New-Object System.Drawing.Size(325,270)
$gleich.Size = New-Object System.Drawing.Size(100,50)
$gleich.Text = "="
$gleich.Name = "="
$Taschenrechner.Controls.Add($gleich)
#Durch
$durch = New-Object System.Windows.Forms.Button
$durch.Location = New-Object System.Drawing.Size(425,120)
$durch.Size = New-Object System.Drawing.Size(100,50)
$durch.Text = "/"
$durch.Name = "/"
$Taschenrechner.Controls.Add($durch)
#Mal
$mal = New-Object System.Windows.Forms.Button
$mal.Location = New-Object System.Drawing.Size(425,170)
$mal.Size = New-Object System.Drawing.Size(100,50)
$mal.Text = "×"
$mal.Name = "*"
$Taschenrechner.Controls.Add($mal)
#Minus
$minus = New-Object System.Windows.Forms.Button
$minus.Location = New-Object System.Drawing.Size(425,220)
$minus.Size = New-Object System.Drawing.Size(100,50)
$minus.Text = "-"
$minus.Name = "-"
$Taschenrechner.Controls.Add($minus)
#Plus
$plus = New-Object System.Windows.Forms.Button
$plus.Location = New-Object System.Drawing.Size(425,270)
$plus.Size = New-Object System.Drawing.Size(100,50)
$plus.Text = "+"
$plus.Name = "+"
$Taschenrechner.Controls.Add($plus)
Function Operation {
param([string] $Ope)
### you need to show partial result an operation!!!
if ( $Textfeld.Text -ne '' ) {
if ($script:PartialResult -ne '' ) {
$aux = [string] $script:PartialResult + $script:PartOperation + $script:Textfeld.Text
$script:PartialResult = [string] $( Invoke-Expression $aux )
} else {
$script:PartialResult = $Textfeld.Text
}
if ( $Ope -eq '=' ) {
$Textfeld.Text = '' + [string] $script:PartialResult
$script:PartialResult = ''
$script:PartOperation = ''
} else {
$Textfeld.Text = ''
$script:PartOperation = $Ope
}
}
}
$gleich.Add_Click( { Operation '=' } )
$mal. Add_Click( { Operation '*' } ) # Name = "×"
$plus. Add_Click( { Operation '+' } ) # Name = "+"
$minus. Add_Click( { Operation '-' } ) # Name = "-"
$durch. Add_Click( { Operation '/' } ) # Name = "/"
[void] $Taschenrechner.ShowDialog()
Hello JosefZ thanks for your answer. Could you explain me what the Funtion Operation exactly is doing?
– Sandro21
Jan 22 '17 at 11:43
add a comment |
The following improvement of my previous answer to your almost the same question could help however such patchwork would conduce you to perdition (as well as me). Good luck!
Remove-Variable script:Part* -ErrorAction SilentlyContinue
$script:PartialResult = ''
$script:PartOperation = ''
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Drawing")
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
#Platfrom
### unchanged code here (cca 110 lines)
#Dezimalstelle
$Punkt = New-Object System.Windows.Forms.Button
$Punkt.Location = New-Object System.Drawing.Size(225,270)
$Punkt.Size = New-Object System.Drawing.Size(100,50)
$Punkt.Add_Click({$Textfeld.Text+='.'})
$Punkt.Text = "."
$Punkt.Name = "."
$Taschenrechner.Controls.Add($Punkt)
#Gleich
$gleich = New-Object System.Windows.Forms.Button
$gleich.Location = New-Object System.Drawing.Size(325,270)
$gleich.Size = New-Object System.Drawing.Size(100,50)
$gleich.Text = "="
$gleich.Name = "="
$Taschenrechner.Controls.Add($gleich)
#Durch
$durch = New-Object System.Windows.Forms.Button
$durch.Location = New-Object System.Drawing.Size(425,120)
$durch.Size = New-Object System.Drawing.Size(100,50)
$durch.Text = "/"
$durch.Name = "/"
$Taschenrechner.Controls.Add($durch)
#Mal
$mal = New-Object System.Windows.Forms.Button
$mal.Location = New-Object System.Drawing.Size(425,170)
$mal.Size = New-Object System.Drawing.Size(100,50)
$mal.Text = "×"
$mal.Name = "*"
$Taschenrechner.Controls.Add($mal)
#Minus
$minus = New-Object System.Windows.Forms.Button
$minus.Location = New-Object System.Drawing.Size(425,220)
$minus.Size = New-Object System.Drawing.Size(100,50)
$minus.Text = "-"
$minus.Name = "-"
$Taschenrechner.Controls.Add($minus)
#Plus
$plus = New-Object System.Windows.Forms.Button
$plus.Location = New-Object System.Drawing.Size(425,270)
$plus.Size = New-Object System.Drawing.Size(100,50)
$plus.Text = "+"
$plus.Name = "+"
$Taschenrechner.Controls.Add($plus)
Function Operation {
param([string] $Ope)
### you need to show partial result an operation!!!
if ( $Textfeld.Text -ne '' ) {
if ($script:PartialResult -ne '' ) {
$aux = [string] $script:PartialResult + $script:PartOperation + $script:Textfeld.Text
$script:PartialResult = [string] $( Invoke-Expression $aux )
} else {
$script:PartialResult = $Textfeld.Text
}
if ( $Ope -eq '=' ) {
$Textfeld.Text = '' + [string] $script:PartialResult
$script:PartialResult = ''
$script:PartOperation = ''
} else {
$Textfeld.Text = ''
$script:PartOperation = $Ope
}
}
}
$gleich.Add_Click( { Operation '=' } )
$mal. Add_Click( { Operation '*' } ) # Name = "×"
$plus. Add_Click( { Operation '+' } ) # Name = "+"
$minus. Add_Click( { Operation '-' } ) # Name = "-"
$durch. Add_Click( { Operation '/' } ) # Name = "/"
[void] $Taschenrechner.ShowDialog()
The following improvement of my previous answer to your almost the same question could help however such patchwork would conduce you to perdition (as well as me). Good luck!
Remove-Variable script:Part* -ErrorAction SilentlyContinue
$script:PartialResult = ''
$script:PartOperation = ''
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Drawing")
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
#Platfrom
### unchanged code here (cca 110 lines)
#Dezimalstelle
$Punkt = New-Object System.Windows.Forms.Button
$Punkt.Location = New-Object System.Drawing.Size(225,270)
$Punkt.Size = New-Object System.Drawing.Size(100,50)
$Punkt.Add_Click({$Textfeld.Text+='.'})
$Punkt.Text = "."
$Punkt.Name = "."
$Taschenrechner.Controls.Add($Punkt)
#Gleich
$gleich = New-Object System.Windows.Forms.Button
$gleich.Location = New-Object System.Drawing.Size(325,270)
$gleich.Size = New-Object System.Drawing.Size(100,50)
$gleich.Text = "="
$gleich.Name = "="
$Taschenrechner.Controls.Add($gleich)
#Durch
$durch = New-Object System.Windows.Forms.Button
$durch.Location = New-Object System.Drawing.Size(425,120)
$durch.Size = New-Object System.Drawing.Size(100,50)
$durch.Text = "/"
$durch.Name = "/"
$Taschenrechner.Controls.Add($durch)
#Mal
$mal = New-Object System.Windows.Forms.Button
$mal.Location = New-Object System.Drawing.Size(425,170)
$mal.Size = New-Object System.Drawing.Size(100,50)
$mal.Text = "×"
$mal.Name = "*"
$Taschenrechner.Controls.Add($mal)
#Minus
$minus = New-Object System.Windows.Forms.Button
$minus.Location = New-Object System.Drawing.Size(425,220)
$minus.Size = New-Object System.Drawing.Size(100,50)
$minus.Text = "-"
$minus.Name = "-"
$Taschenrechner.Controls.Add($minus)
#Plus
$plus = New-Object System.Windows.Forms.Button
$plus.Location = New-Object System.Drawing.Size(425,270)
$plus.Size = New-Object System.Drawing.Size(100,50)
$plus.Text = "+"
$plus.Name = "+"
$Taschenrechner.Controls.Add($plus)
Function Operation {
param([string] $Ope)
### you need to show partial result an operation!!!
if ( $Textfeld.Text -ne '' ) {
if ($script:PartialResult -ne '' ) {
$aux = [string] $script:PartialResult + $script:PartOperation + $script:Textfeld.Text
$script:PartialResult = [string] $( Invoke-Expression $aux )
} else {
$script:PartialResult = $Textfeld.Text
}
if ( $Ope -eq '=' ) {
$Textfeld.Text = '' + [string] $script:PartialResult
$script:PartialResult = ''
$script:PartOperation = ''
} else {
$Textfeld.Text = ''
$script:PartOperation = $Ope
}
}
}
$gleich.Add_Click( { Operation '=' } )
$mal. Add_Click( { Operation '*' } ) # Name = "×"
$plus. Add_Click( { Operation '+' } ) # Name = "+"
$minus. Add_Click( { Operation '-' } ) # Name = "-"
$durch. Add_Click( { Operation '/' } ) # Name = "/"
[void] $Taschenrechner.ShowDialog()
edited Mar 20 '17 at 10:04
Community♦
1
1
answered Jan 21 '17 at 20:25


JosefZJosefZ
7,51041544
7,51041544
Hello JosefZ thanks for your answer. Could you explain me what the Funtion Operation exactly is doing?
– Sandro21
Jan 22 '17 at 11:43
add a comment |
Hello JosefZ thanks for your answer. Could you explain me what the Funtion Operation exactly is doing?
– Sandro21
Jan 22 '17 at 11:43
Hello JosefZ thanks for your answer. Could you explain me what the Funtion Operation exactly is doing?
– Sandro21
Jan 22 '17 at 11:43
Hello JosefZ thanks for your answer. Could you explain me what the Funtion Operation exactly is doing?
– Sandro21
Jan 22 '17 at 11:43
add a comment |
Thanks for contributing an answer to Super User!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsuperuser.com%2fquestions%2f1170043%2fcreate-a-calculator-with-powershell-gui%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8 ifeiXXlFDHnX5N,IaVlBGqomoHef 2kuGpiKSx4ZJsTfkACtFoB,w2mXRKgvh,kw4c1sZ3nU8 TyX7Asf91XyyvEEtf
1
If you're pulling those stunts with PowerShell just go ahead and write a "real" C# Program. it's going to be less of a headache and using Visual Studio would mean you would have a lot of GUI help for a lot of things (IntelliSense etc.). What you would need to do is bind to the event for the buttons and let the function you bind to the event change the text for the input.
– Seth
Jan 21 '17 at 15:24