Singleton set manager in c++ [on hold]
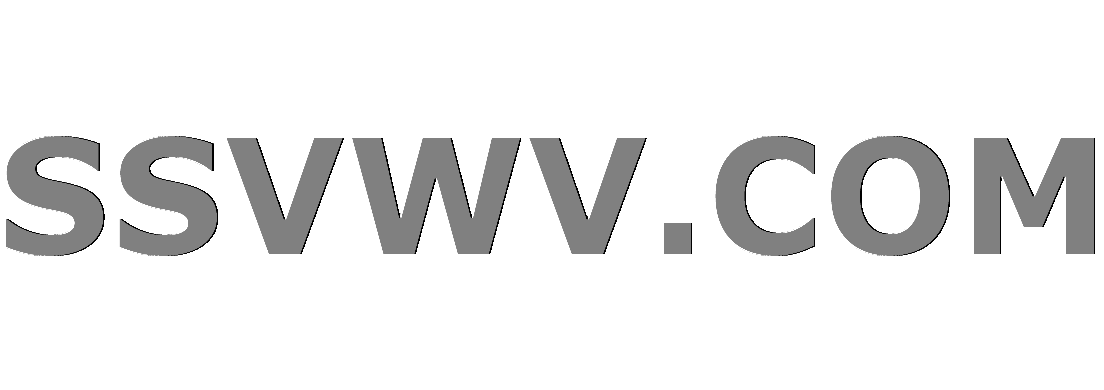
Multi tool use
$begingroup$
I thought a singleton set
manager is better than a global set
. So I wrote a singleton set manager class C
.
Minimal requirement of the class C
- must have insert() to insert a new item(int)
- must have has() to check if it has the item
- should be a singleton
Questions
- I wonder this is a good singleton implementation or not.
- How can I make this code better?
Code
#include <iostream>
#include <iterator>
#include <set>
#include <vector>
#include <initializer_list>
class C {
std::set<int> s;
public:
static C &getInstance() {
static C instance;
return instance;
}
bool insert(int i) {
std::pair<std::set<int>::iterator, bool> ret = s.insert(i);
return ret.second;
}
bool has(int i) {
return s.find(i) != s.end();
}
/* dump() is not essential */
void dump() {
std::copy(s.begin(), s.end(),
std::ostream_iterator<int>(std::cout, " "));
std::cout << "n";
}
private:
C() {}
/* prevent to call C(C const &) */
C(C const &);
/* prevent to call operator=() */
void operator=(C const &);
};
void foo(std::vector<int> &v) {
C &c = C::getInstance();
for (int i = 0; i < v.size(); i++) {
c.insert(v[i]);
}
}
void bar(std::vector<int> &v) {
C &c = C::getInstance();
for (int i = 0; i < v.size(); i++) {
std::cout << v[i] << " " << (c.has(v[i]) ? "y " : "n ");
}
std::cout << "n";
}
int main() {
std::vector<int> x{1, 3, 5, 7};
std::vector<int> y{1, 4};
std::vector<int> z{3, 4, 10};
C &c = C::getInstance();
c.dump();
foo(x);
c.dump();
bar(z);
foo(y);
c.dump();
bar(z);
}
Output
dump:
dump: 1 3 5 7
3 y 4 n 10 n
dump: 1 3 4 5 7
3 y 4 y 10 n
c++ beginner singleton
$endgroup$
put on hold as off-topic by Jamal♦ 2 hours ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
$begingroup$
I thought a singleton set
manager is better than a global set
. So I wrote a singleton set manager class C
.
Minimal requirement of the class C
- must have insert() to insert a new item(int)
- must have has() to check if it has the item
- should be a singleton
Questions
- I wonder this is a good singleton implementation or not.
- How can I make this code better?
Code
#include <iostream>
#include <iterator>
#include <set>
#include <vector>
#include <initializer_list>
class C {
std::set<int> s;
public:
static C &getInstance() {
static C instance;
return instance;
}
bool insert(int i) {
std::pair<std::set<int>::iterator, bool> ret = s.insert(i);
return ret.second;
}
bool has(int i) {
return s.find(i) != s.end();
}
/* dump() is not essential */
void dump() {
std::copy(s.begin(), s.end(),
std::ostream_iterator<int>(std::cout, " "));
std::cout << "n";
}
private:
C() {}
/* prevent to call C(C const &) */
C(C const &);
/* prevent to call operator=() */
void operator=(C const &);
};
void foo(std::vector<int> &v) {
C &c = C::getInstance();
for (int i = 0; i < v.size(); i++) {
c.insert(v[i]);
}
}
void bar(std::vector<int> &v) {
C &c = C::getInstance();
for (int i = 0; i < v.size(); i++) {
std::cout << v[i] << " " << (c.has(v[i]) ? "y " : "n ");
}
std::cout << "n";
}
int main() {
std::vector<int> x{1, 3, 5, 7};
std::vector<int> y{1, 4};
std::vector<int> z{3, 4, 10};
C &c = C::getInstance();
c.dump();
foo(x);
c.dump();
bar(z);
foo(y);
c.dump();
bar(z);
}
Output
dump:
dump: 1 3 5 7
3 y 4 n 10 n
dump: 1 3 4 5 7
3 y 4 y 10 n
c++ beginner singleton
$endgroup$
put on hold as off-topic by Jamal♦ 2 hours ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
$begingroup$
I thought a singleton set
manager is better than a global set
. So I wrote a singleton set manager class C
.
Minimal requirement of the class C
- must have insert() to insert a new item(int)
- must have has() to check if it has the item
- should be a singleton
Questions
- I wonder this is a good singleton implementation or not.
- How can I make this code better?
Code
#include <iostream>
#include <iterator>
#include <set>
#include <vector>
#include <initializer_list>
class C {
std::set<int> s;
public:
static C &getInstance() {
static C instance;
return instance;
}
bool insert(int i) {
std::pair<std::set<int>::iterator, bool> ret = s.insert(i);
return ret.second;
}
bool has(int i) {
return s.find(i) != s.end();
}
/* dump() is not essential */
void dump() {
std::copy(s.begin(), s.end(),
std::ostream_iterator<int>(std::cout, " "));
std::cout << "n";
}
private:
C() {}
/* prevent to call C(C const &) */
C(C const &);
/* prevent to call operator=() */
void operator=(C const &);
};
void foo(std::vector<int> &v) {
C &c = C::getInstance();
for (int i = 0; i < v.size(); i++) {
c.insert(v[i]);
}
}
void bar(std::vector<int> &v) {
C &c = C::getInstance();
for (int i = 0; i < v.size(); i++) {
std::cout << v[i] << " " << (c.has(v[i]) ? "y " : "n ");
}
std::cout << "n";
}
int main() {
std::vector<int> x{1, 3, 5, 7};
std::vector<int> y{1, 4};
std::vector<int> z{3, 4, 10};
C &c = C::getInstance();
c.dump();
foo(x);
c.dump();
bar(z);
foo(y);
c.dump();
bar(z);
}
Output
dump:
dump: 1 3 5 7
3 y 4 n 10 n
dump: 1 3 4 5 7
3 y 4 y 10 n
c++ beginner singleton
$endgroup$
I thought a singleton set
manager is better than a global set
. So I wrote a singleton set manager class C
.
Minimal requirement of the class C
- must have insert() to insert a new item(int)
- must have has() to check if it has the item
- should be a singleton
Questions
- I wonder this is a good singleton implementation or not.
- How can I make this code better?
Code
#include <iostream>
#include <iterator>
#include <set>
#include <vector>
#include <initializer_list>
class C {
std::set<int> s;
public:
static C &getInstance() {
static C instance;
return instance;
}
bool insert(int i) {
std::pair<std::set<int>::iterator, bool> ret = s.insert(i);
return ret.second;
}
bool has(int i) {
return s.find(i) != s.end();
}
/* dump() is not essential */
void dump() {
std::copy(s.begin(), s.end(),
std::ostream_iterator<int>(std::cout, " "));
std::cout << "n";
}
private:
C() {}
/* prevent to call C(C const &) */
C(C const &);
/* prevent to call operator=() */
void operator=(C const &);
};
void foo(std::vector<int> &v) {
C &c = C::getInstance();
for (int i = 0; i < v.size(); i++) {
c.insert(v[i]);
}
}
void bar(std::vector<int> &v) {
C &c = C::getInstance();
for (int i = 0; i < v.size(); i++) {
std::cout << v[i] << " " << (c.has(v[i]) ? "y " : "n ");
}
std::cout << "n";
}
int main() {
std::vector<int> x{1, 3, 5, 7};
std::vector<int> y{1, 4};
std::vector<int> z{3, 4, 10};
C &c = C::getInstance();
c.dump();
foo(x);
c.dump();
bar(z);
foo(y);
c.dump();
bar(z);
}
Output
dump:
dump: 1 3 5 7
3 y 4 n 10 n
dump: 1 3 4 5 7
3 y 4 y 10 n
c++ beginner singleton
c++ beginner singleton
asked 3 hours ago


H. JangH. Jang
675
675
put on hold as off-topic by Jamal♦ 2 hours ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
put on hold as off-topic by Jamal♦ 2 hours ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
add a comment |
0
active
oldest
votes
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
hSD MzpTQ4Y2SGcJCeMBvn5c2sy PsC2ebzLgpyDnaYP8g7el TvktgO sAlEy7,GE dK1YjGHAqp7cfKd49qfbB 6iYdR